在vue 中,如何跳转到tabBar 页面,给个例子
时间: 2023-08-31 17:33:26 浏览: 62
### 回答1:
在 Vue 中,要跳转到一个 TabBar 页面,需要使用 Vue Router,并结合 TabBar 组件实现。以下是一个例子:
```html
<template>
<div>
<tab-bar :selected="selectedTab" @update:selected="selectedTab = $event">
<tab-bar-item name="home" icon="home">首页</tab-bar-item>
<tab-bar-item name="about" icon="info">关于</tab-bar-item>
<tab-bar-item name="contact" icon="message">联系我们</tab-bar-item>
</tab-bar>
<router-view />
</div>
</template>
<script>
import TabBar from "@/components/TabBar";
import TabBarItem from "@/components/TabBarItem";
export default {
name: "App",
components: {
TabBar,
TabBarItem,
},
data() {
return {
selectedTab: "home",
};
},
};
</script>
```
在这个例子中,我们使用了一个 `TabBar` 组件和三个 `TabBarItem` 组件。`TabBar` 组件用于显示整个 TabBar,而 `TabBarItem` 组件用于表示每个 TabBar 的项。
在组件的 `<script>` 标签中,我们使用了 Vue Router,并在 `data` 中定义了 `selectedTab` 属性,用于指示当前选中的 TabBar 项的名称。我们还定义了 `TabBar` 和 `TabBarItem` 组件,并将它们注册到组件中。
现在,我们需要使用 Vue Router 将每个 TabBar 项映射到相应的路由。我们可以在 Vue Router 的配置文件中添加以下路由:
```js
import Vue from "vue";
import VueRouter from "vue-router";
import Home from "@/views/Home.vue";
import About from "@/views/About.vue";
import Contact from "@/views/Contact.vue";
Vue.use(VueRouter);
const routes = [
{
path: "/",
name: "Home",
component: Home,
},
{
path: "/about",
name: "About",
component: About,
},
{
path: "/contact",
name: "Contact",
component: Contact,
},
];
const router = new VueRouter({
mode: "history",
base: process.env.BASE_URL,
routes,
});
export default router;
```
在这个例子中,我们定义了三个路由,分别对应于 `Home`、`About` 和 `Contact` 组件。现在,当用户点击 TabBar 中的任何一个项时,我们需要将用户重定向到相应的路由。为此,我们需要在 `TabBarItem` 组件中添加点击事件:
```html
<template>
<div :class="classes" @click="navigate">
<slot></slot>
</div>
</template>
<script>
export default {
name: "TabBarItem",
props: {
name: {
type: String,
required: true,
},
icon: {
type: String,
required: true,
},
},
computed: {
classes() {
return {
"tab-bar-item": true,
"tab-bar-item-selected": this.isSelected,
};
},
isSelected() {
return this.$parent.selected === this.name;
},
},
methods: {
navigate() {
this.$router.push({ name: this.name });
this.$parent.$emit
### 回答2:
在Vue中,要跳转到TabBar页面,可以使用Vue Router来实现。下面给出一个例子:
首先,在main.js文件中引入Vue和Vue Router,并创建Vue实例和路由实例:
```javascript
import Vue from 'vue'
import VueRouter from 'vue-router'
import App from './App.vue'
import TabBarPage from './components/TabBarPage.vue'
Vue.use(VueRouter)
const routes = [
{ path: '/', component: TabBarPage }
]
const router = new VueRouter({
routes
})
new Vue({
router,
render: h => h(App)
}).$mount('#app')
```
然后,在App.vue组件中添加TabBar导航栏和路由占位符:
```html
<template>
<div id="app">
<tab-bar></tab-bar>
<router-view></router-view>
</div>
</template>
```
接下来,创建一个TabBarPage.vue组件作为TabBar页面的内容:
```html
<template>
<div>
<!-- TabBar页面内容 -->
</div>
</template>
```
最后,在TabBar组件中添加路由链接:
```html
<template>
<div>
<router-link to="/">TabBar页面</router-link>
<!-- 其他TabBar链接 -->
</div>
</template>
```
这样,当点击TabBar组件中的路由链接时,就可以跳转到TabBar页面,显示TabBar页面的内容。
### 回答3:
在Vue中,跳转到TabBar页面可以通过路由配合TabBar组件来实现。下面是一个例子。
首先,在Vue项目的路由配置文件中,定义TabBar页面的路由:
```javascript
import Vue from 'vue'
import VueRouter from 'vue-router'
Vue.use(VueRouter)
const routes = [
{
path: '/',
redirect: '/home'
},
{
path: '/home',
name: 'Home',
component: () => import('@/views/Home.vue')
},
{
path: '/profile',
name: 'Profile',
component: () => import('@/views/Profile.vue')
},
{
path: '/settings',
name: 'Settings',
component: () => import('@/views/Settings.vue')
}
]
const router = new VueRouter({
routes
})
export default router
```
然后,在主页面(App.vue)中,引入TabBar组件,将路由链接与TabBar标签项对应起来:
```html
<template>
<div>
<router-view></router-view>
<tab-bar>
<tab-bar-item to="/home">首页</tab-bar-item>
<tab-bar-item to="/profile">个人资料</tab-bar-item>
<tab-bar-item to="/settings">设置</tab-bar-item>
</tab-bar>
</div>
</template>
<script>
import TabBar from '@/components/TabBar.vue'
import TabBarItem from '@/components/TabBarItem.vue'
export default {
name: 'App',
components: {
TabBar,
TabBarItem
}
}
</script>
```
最后,在TabBar组件(TabBar.vue)中,定义链接的样式和路由跳转的功能:
```html
<template>
<div class="tab-bar">
<slot></slot>
</div>
</template>
<script>
export default {
name: 'TabBar'
}
</script>
<style scoped>
.tab-bar {
position: fixed;
bottom: 0;
left: 0;
right: 0;
height: 50px;
background-color: #fff;
border-top: 1px solid #eee;
display: flex;
}
.tab-bar-item {
flex: 1;
display: flex;
align-items: center;
justify-content: center;
font-size: 14px;
color: #999;
}
.tab-bar-item.active {
color: #007aff;
}
</style>
```
在TabBarItem组件(TabBarItem.vue)中,为链接添加点击事件,实现路由跳转:
```html
<template>
<div :class="['tab-bar-item', { active: isActive }]">
<router-link :to="to" @click.native="handleClick">
<slot></slot>
</router-link>
</div>
</template>
<script>
export default {
name: 'TabBarItem',
props: {
to: {
type: String,
required: true
}
},
computed: {
isActive() {
return this.$route.path === this.to
}
},
methods: {
handleClick() {
this.$router.push(this.to)
}
}
}
</script>
<style scoped>
.tab-bar-item.active {
color: #007aff;
}
</style>
```
以上是一个简单的例子,通过路由和TabBar组件,可以实现在Vue中跳转到TabBar页面。
相关推荐
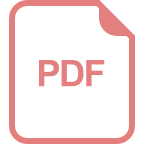
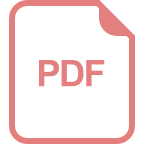














