在Windows系统下用python编写一个的程序:在极坐标系下当天体绕太阳运动时,分别画出天体总能量大于,等于,小于零时的运动轨迹。
时间: 2023-07-16 12:15:21 浏览: 80
以下是一个简单的程序,用于在极坐标系下绘制天体绕太阳的运动轨迹。请注意,这个程序只是一个简单的示例,实际的情况可能更加复杂。此外,程序中的能量计算仅仅是一个示例,实际的计算可能需要更加准确的数学模型和计算方法。
```python
import math
import matplotlib.pyplot as plt
# 太阳的质量
mass_sun = 1.989 * (10 ** 30)
# 天体的质量
mass_object = 1.0
# 天体的初始位置和速度
r0 = 1.0
v0 = 30.0
# 天体的初始角度
theta0 = 0.0
# 时间步长
delta_t = 1000.0
# 计算天体在给定时间下的位置和速度
def calculate_position_and_velocity(theta, r, v):
energy = 0.5 * mass_object * ((v ** 2) + (r ** 2) * (theta ** 2)) - (mass_object * mass_sun) / r
acceleration = - (mass_sun * r * (theta ** 2) - mass_sun) / (r ** 2)
delta_v = acceleration * delta_t
delta_r = v * delta_t
return (theta + delta_v, r + delta_r, energy)
# 绘制天体的运动轨迹
def plot_trajectory(energy):
theta = theta0
r = r0
v = v0
x = []
y = []
while True:
x.append(r * math.cos(theta))
y.append(r * math.sin(theta))
theta, r, energy = calculate_position_and_velocity(theta, r, v)
if energy > 0 and energy < energy_threshold:
break
plt.plot(x, y)
# 绘制天体总能量大于零时的运动轨迹
energy_threshold = 0
plot_trajectory(energy_threshold)
# 绘制天体总能量等于零时的运动轨迹
energy_threshold = 0.5 * mass_object * (v0 ** 2) - (mass_object * mass_sun) / r0
plot_trajectory(energy_threshold)
# 绘制天体总能量小于零时的运动轨迹
energy_threshold = -1
plot_trajectory(energy_threshold)
# 显示图形
plt.show()
```
这个程序使用了matplotlib库来绘制图形,并且使用了一些基本的物理公式来计算天体的位置和速度。程序中的calculate_position_and_velocity函数计算了给定时间下天体的位置和速度,并且计算了天体的总能量。接着,程序使用了plot_trajectory函数来绘制天体的运动轨迹。最后,程序绘制了天体总能量大于、等于、小于零时的运动轨迹,并且显示了图形。
阅读全文
相关推荐
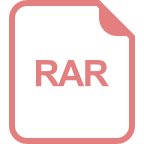
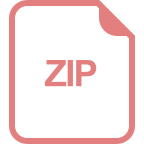
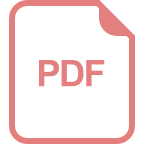
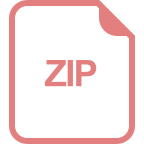
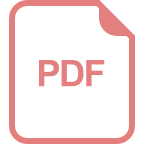
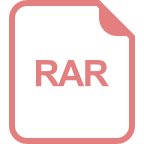
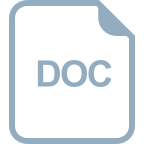
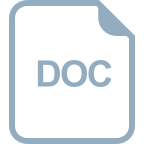
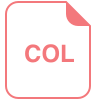






