python布局子图,进行子图填充
时间: 2023-07-25 15:26:06 浏览: 57
要进行Python布局子图并进行子图填充,可以使用Matplotlib库。以下是一个示例代码:
```python
import matplotlib.pyplot as plt
# 创建主图和子图
fig, axs = plt.subplots(2, 2)
# 绘制子图1
axs[0, 0].plot([1, 2, 3], [2, 4, 3])
axs[0, 0].set_title('Subplot 1')
# 绘制子图2
axs[0, 1].scatter([1, 2, 3], [2, 4, 3])
axs[0, 1].set_title('Subplot 2')
# 绘制子图3
axs[1, 0].hist([1, 2, 2, 3, 3, 3])
axs[1, 0].set_title('Subplot 3')
# 绘制子图4
axs[1, 1].pie([1, 2, 2, 3, 3, 3])
axs[1, 1].set_title('Subplot 4')
# 调整子图间距和边距
fig.subplots_adjust(hspace=0.4, wspace=0.4, left=0.1, right=0.9, top=0.9, bottom=0.1)
# 显示图形
plt.show()
```
在这个例子中,我们创建了一个2x2的子图网格,并在每个子图中绘制了不同类型的图形。我们还使用`subplots_adjust()`函数调整了子图之间的间距和边距。最后,我们使用`show()`函数显示图形。
相关问题
pythonmatplotlib库
Python的Matplotlib库是一个非常强大的数据可视化工具,它提供了一套丰富的图形绘制功能,可以创建静态、动态以及交互式的图表。Matplotlib支持线图、散点图、柱状图、饼图、直方图等各种类型的图表,并且它的API设计灵活,能够满足各种复杂的绘图需求。
使用Matplotlib,你可以通过简单的代码快速生成图表,例如导入数据,设置图表标题、坐标轴标签、样式等基本元素,然后调用相应函数绘制线条、填充区域或添加图例。此外,还有一些高级特性,如子图(subplot)布局、保存图像文件、添加注解等。
如果你是一名Python开发者,无论是数据分析、科学计算还是机器学习项目,Matplotlib都是不可或缺的数据可视化伙伴。要开始使用Matplotlib,需要先安装它,通常通过pip install matplotlib命令即可完成。
python 删除空白子图
要删除空白子图,可以使用 `del` 关键字删除 `ax` 对象。删除空白子图后,可以使用 `subplots_adjust()` 函数调整子图之间的间距,以填充删除的子图的空白位置。
以下是一个示例代码,演示如何删除空白子图:
```python
import matplotlib.pyplot as plt
# 创建一个包含多个子图的图形,并获取每个子图的 ax 对象
fig, axs = plt.subplots(2, 2)
# 删除第四个子图
del axs[1, 1]
# 调整子图之间的间距
plt.subplots_adjust(wspace=0.4, hspace=0.4)
# 显示图形
plt.show()
```
在上述代码中,我们创建了一个包含2行2列的子图的图形,并将每个子图的 `ax` 对象存储在 `axs` 数组中。然后,我们使用 `del` 关键字删除了第四个子图的 `ax` 对象。最后,我们使用 `subplots_adjust()` 函数调整子图之间的间距。
通过删除空白子图并调整间距,我们可以使其他子图填充空白子图的位置,以保持整体布局的一致性。
希望这个示例对你有帮助。如果你有任何进一步的问题,请随时提问。
相关推荐
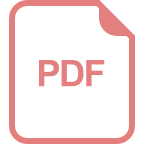
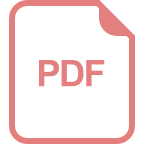








