执行#include <stdio.h> #include <pthread.h> #include <time.h> int x=0; int y=0; void* a() { x=1; y=y+x; sleep(0.01); printf("x=%d\ty=%d\n",x,y); return NULL; } void* b() { y=4; x=5+x; sleep(0.02); printf("x=%d\ty=%d\n",x,y); return NULL; } int main() { pthread_t A,B; pthread_create(&A,NULL,a,NULL); pthread_create(&B,NULL,b,NULL); pthread_join(A,NULL); pthread_join(B,NULL); printf("x=%d\ty=%d\n",x,y); return 0; }
时间: 2024-01-18 09:03:09 浏览: 59
这是一个使用 pthread 库创建多线程的 C 语言程序。该程序创建了两个线程 A 和 B,分别执行函数 a 和函数 b。
在函数 a 中,将全局变量 x 赋值为 1,然后将 y 的值加上 x 的值,即 y = y + x。随后程序暂停 0.01 秒,最后输出 x 和 y 的值。
在函数 b 中,将全局变量 y 赋值为 4,然后将 x 的值加上 5 加上 x 的值,即 x = 5 + x。随后程序暂停 0.02 秒,最后输出 x 和 y 的值。
在主函数中,程序先创建了线程 A 和线程 B,然后等待线程 A 和线程 B 执行完毕。最后输出 x 和 y 的值。
需要注意的是,由于线程 A 和线程 B 的执行是并发的,因此最终输出的 x 和 y 的值可能不同于预期值,因为线程的执行顺序是不确定的。
相关问题
以下代码有什么作用,#include <pthread.h> #include <stdio.h> #include <unistd.h> volatile unsigned long lock = 0; void my_lock( volatile unsigned long * lock ) { } void my_unlock( volatile unsigned long * lock ) { } int count; void increment( int ntimes ) {
这段代码中包含了线程相关的头文件和一些变量的定义。具体解释如下:
- `#include <pthread.h>`:这个头文件包含了创建和管理线程的相关函数和数据类型。
- `#include <stdio.h>`:这个头文件包含了输入输出函数。
- `#include <unistd.h>`:这个头文件包含了一些系统调用函数,例如 `sleep()`。
接下来定义了一个全局变量 `lock`,它是一个无符号长整型,并且被声明为 `volatile`。`volatile` 关键字告诉编译器该变量的值可能会在程序执行期间发生变化,因此不应该对此变量进行优化。
然后定义了两个函数 `my_lock()` 和 `my_unlock()`,这两个函数的实现并没有给出,只是声明了函数原型。这两个函数的作用是获取和释放锁。
接下来定义了一个整型变量 `count`,并初始化为 0。
最后有一个函数 `increment()`,它接受一个整数参数 `ntimes`,表示需要增加计数器的次数。函数体中实现了一个循环,每次循环先获取锁,然后将计数器加 1,最后释放锁。循环的次数由 `ntimes` 决定。
补全代码#include <stdio.h> #include <stdlib.h> #include <string.h> #include <errno.h> #include <unistd.h> #include <sys/types.h> #include <sys/socket.h> #include <sys/shm.h> #include <pthread.h> #include "mypthread.h" #include "serial.h" #include "zigbee.h" #include "my_camera.h" #include "server.h" static int zgbfd; static pthread_mutex_t cam_mutex; void *pthread_cam(void *arg) { } void *pthread_zgb(void *arg) { } void *pthread_cli(void *arg) { }
下面是对代码的补全:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <errno.h>
#include <unistd.h>
#include <sys/types.h>
#include <sys/socket.h>
#include <sys/shm.h>
#include <pthread.h>
#include "mypthread.h"
#include "serial.h"
#include "zigbee.h"
#include "my_camera.h"
#include "server.h"
static int zgbfd;
static pthread_mutex_t cam_mutex;
void *pthread_cam(void *arg)
{
// 摄像头相关操作的具体实现
while (1) {
// 在这里实现摄像头的操作逻辑
// 例如获取摄像头图像并处理
// 使用互斥锁保护临界区
pthread_mutex_lock(&cam_mutex);
// 执行摄像头操作
pthread_mutex_unlock(&cam_mutex);
// 休眠一段时间
usleep(1000);
}
return NULL;
}
void *pthread_zgb(void *arg)
{
// Zigbee 相关操作的具体实现
while (1) {
// 在这里实现 Zigbee 的操作逻辑
// 例如发送或接收数据
// 使用互斥锁保护临界区
pthread_mutex_lock(&cam_mutex);
// 执行 Zigbee 操作
pthread_mutex_unlock(&cam_mutex);
// 休眠一段时间
usleep(1000);
}
return NULL;
}
void *pthread_cli(void *arg)
{
// 客户端连接和通信操作的具体实现
int client_sock = *((int*)arg);
char buffer[1024];
while (1) {
// 在这里实现客户端连接和通信的操作逻辑
// 例如接收客户端消息并处理
memset(buffer, 0, sizeof(buffer));
int ret = read(client_sock, buffer, sizeof(buffer));
if (ret <= 0) {
// 客户端断开连接或出错,结束线程
close(client_sock);
break;
}
// 处理客户端消息
}
return NULL;
}
```
以上补全了 `pthread_cam`、`pthread_zgb` 和 `pthread_cli` 函数的代码实现部分。你可以根据具体需求在这些函数中添加逻辑来完成相应的操作。注意使用互斥锁来保护临界区,以避免多个线程同时访问造成的竞争条件。
阅读全文
相关推荐
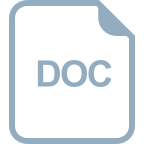
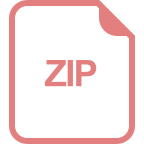
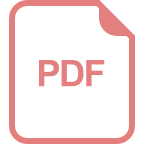














