用C++编写程序读入一段文本到vector对象,每个单词存储为vector中的一个元素。把vector对象中每个单词转化为大写字母。输出vector对象中转化后的元素,每八个单词为一行输出。
时间: 2024-05-04 18:16:16 浏览: 104
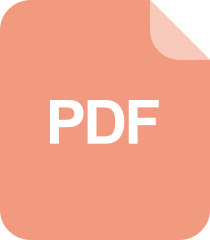
用c++实现将文本每个单词首字母转换为大写

好的,让我来回答你的问题。你可以按照以下代码,使用C++编写程序读取文本并将单词存储到vector对象中。然后,可以使用for循环将个单词转换为大写字母,并将它们打印出来,每八个单词为一行输出。
```
#include <iostream>
#include <vector>
#include <string>
#include <algorithm>
using namespace std;
int main() {
vector<string> words;
string word;
while (cin >> word) {
words.push_back(word);
}
for (int i = 0; i < words.size(); i++) {
transform(words[i].begin(), words[i].end(), words[i].begin(), ::toupper);
cout << words[i];
if ((i + 1) % 8 == 0) {
cout << endl;
} else {
cout << " ";
}
}
cout << endl;
return 0;
}
```
注意,该程序假定你的输入文本以空格分隔的单词为单位。如果你需要处理其他类型的输入文本,你可能需要调整代码以满足你的要求。
阅读全文
相关推荐
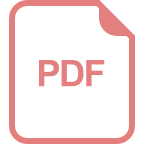








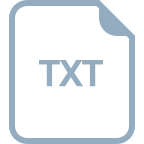





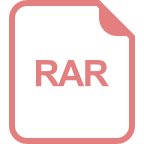