python代码实现:如果pytesseract.image_to_string读不出来数据,则返回''
时间: 2023-03-30 19:03:08 浏览: 118
可以使用try-except语句来实现:
```
import pytesseract
def image_to_string(image):
try:
text = pytesseract.image_to_string(image)
except:
text = ''
return text
```
这样,如果pytesseract.image_to_string无法读取数据,函数将返回一个空字符串''。
相关问题
如何使用pytesseract.image_to_string函数来判断图片是否有文字,不用返回内容,只要有一个文字就可以中断pytesseract.image_to_string程序,返回有文字
由于`pytesseract.image_to_string`是直接返回字符串的函数,无法通过中断程序的方式来返回结果。但是,你可以通过设置`tesseract_cmd`参数来控制`tesseract`命令的执行,进而实现中断程序的目的。
具体来说,你可以在`tesseract_cmd`参数中添加`-psm 0`参数,这个参数的作用是告诉`tesseract`命令,将整个图片作为一个文本块进行识别。这样,如果图片中有文字,`pytesseract.image_to_string`函数会返回一个非空字符串,如果没有文字,则返回一个空字符串。代码示例如下:
```python
import pytesseract
from PIL import Image
img = Image.open('test.png')
config = '--psm 0'
text = pytesseract.image_to_string(img, config=config)
if text:
print('图片中有文字')
# 中断程序,返回有文字
else:
print('图片中没有文字')
```
当程序判断出图片中有文字时,你可以使用`return`语句中断程序并返回结果,或者使用`break`语句跳出循环,具体根据你的应用场景来决定。
Python中pytesseract.image_to_string()的用法
在Python中,`pytesseract.image_to_string()`函数是Pytesseract库的核心部分,用于从图像文件或PIL Image对象中识别出文本内容。它的基本语法如下:
```python
import pytesseract
from PIL import Image
# 加载图像文件
image = Image.open('your_image_path.jpg')
# 使用pytesseract将图像转换为文本字符串
text = pytesseract.image_to_string(image)
# 输出识别到的文本
print(text)
```
这里需要注意几个关键点:
1. 首先,你需要导入所需的模块,即`pytesseract`和`PIL`(Python Imaging Library,也称为Pillow),它们分别负责OCR(光学字符识别)和图像处理。
2. 使用`Image.open()`加载你想要识别的图片,替换 `'your_image_path.jpg'` 为实际的图像文件路径。
3. 调用`pytesseract.image_to_string()`函数,传入刚才加载的图像对象作为参数。
4. 最后,打印识别出的文本结果。
使用这个函数前,请确保已安装必要的库(如`pytesseract`本身以及Tesseract OCR)并且Tesseract在你的系统路径下可用。
阅读全文
相关推荐
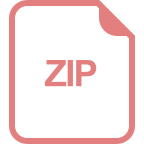
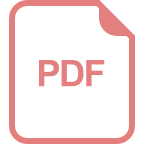













