#include<iostream> #include<string> #include<fstream> using namespace std; const int MAXL = 20; //结点信息 struct Node { string data;//结点值 int no;//结点编号 Node(string data):data(data),no(0){} Node() { data = ""; no = 0; } }; //边结点 struct EdgeNode { Node nextnode;//终止结点信息 EdgeNode* nextedge;//下一条邻接边 EdgeNode(string s) { this->nextnode.data = s; this->nextnode.no = 0; this->nextedge = NULL; } EdgeNode() { this->nextnode.data = ""; this->nextnode.no = 0; this->nextedge = NULL; } }; //表头结点 struct HNode { Node hnode;//起始结点信息 EdgeNode* firstedge;//第一条邻接边 HNode(string s) { hnode.data = s; hnode.no = 0; firstedge = NULL; } HNode() { hnode.data = ""; hnode.no = 0; firstedge = NULL; } }; //邻接表 struct ALGraph { int n, e;//分别表示顶点数和边数 HNode hnode[MAXL];//头结点数组 ALGraph() { n = e = 0; for (int i = 0; i < MAXL; i++) hnode[i].firstedge = NULL; } };
时间: 2024-02-14 18:23:20 浏览: 113
这是一个基于邻接表实现的图的数据结构定义,包括了结点信息、边结点、表头结点和邻接表等。其中,结点信息Node包含了结点的值和编号,边结点EdgeNode包含了终止结点的信息和下一条邻接边,表头结点HNode包含了起始结点的信息和第一条邻接边,邻接表ALGraph则是由多个表头结点组成的数组,用于描述图的拓扑结构。
相关问题
编程实现有向图图的邻接矩阵存储,并计算给定结点的入度和出度。【输入形式】 【输出形式】 【样例输入】(所有数据从键盘输入) 4 4 A B C D A B A D B C C A A 【样例输出】 This graph has 4 vertexs, and 4 edges. The information of vertexs are: A 0 B 1 C 2 D 3 The adjacent matrix of graph is: 0 1 0 1 0 0 1 0 1 0 0 0 0 0 0 0 The in-degree of A is 1 The out-degree of A is 2 #include<iostream> #include<string> #include<cstring> #include<fstream> using namespace std; const int MAXL = 20; struct Node {//结点 string data;//结点值 int no;//结点编号 Node(string data):data(data),no(0){} Node() { data = ""; no = 0; } }; struct MGraph { int n, e;//顶点数和边数 Node VEXS[MAXL];//顶点数组 int Edge[MAXL][MAXL];//邻接矩阵 MGraph() { n = e = 0; memset(Edge, 0, sizeof(Edge)); } };
#include<iostream>
#include<string>
#include<cstring>
#include<fstream>
using namespace std;
const int MAXL = 20;
struct Node{//结点
string data;//结点值
int no;//结点编号
Node(string data):data(data),no(0){}
Node() { data = ""; no = 0; }
};
struct MGraph{
int n, e;//顶点数和边数
Node VEXS[MAXL];//顶点数组
int Edge[MAXL][MAXL];//邻接矩阵
MGraph() {
n = e = 0;
memset(Edge, 0, sizeof(Edge));
}
};
int main() {
MGraph G;
string start, end;
cin >> G.n >> G.e;
for(int i = 0; i < G.n; i++) {//读入结点数据
string data;
cin >> data;
G.VEXS[i] = Node(data);
G.VEXS[i].no = i;
}
for(int i = 0; i < G.e; i++) {//读入边数据
cin >> start >> end;
int s, e;
for(int j = 0; j < G.n; j++) {//找到起点和终点对应的编号
if(G.VEXS[j].data == start) {
s = j;
}
if(G.VEXS[j].data == end) {
e = j;
}
}
G.Edge[s][e] = 1;//在邻接矩阵中标记该边
}
cout << "This graph has " << G.n << " vertexs, and " << G.e << " edges." << endl;
cout << "The information of vertexs are: " << endl;
for(int i = 0; i < G.n; i++) {//输出结点信息
cout << G.VEXS[i].data << " " << i << " ";
}
cout << endl << "The adjacent matrix of graph is: " << endl;
for(int i = 0; i < G.n; i++) {//输出邻接矩阵
for(int j = 0; j < G.n; j++) {
cout << G.Edge[i][j] << " ";
}
cout << endl;
}
string node;
cin >> node;
int no;
for(int i = 0; i < G.n; i++) {//找到指定结点的编号
if(G.VEXS[i].data == node) {
no = i;
break;
}
}
int in_degree = 0, out_degree = 0;
for(int i = 0; i < G.n; i++) {//计算指定结点的入度和出度
if(G.Edge[i][no] == 1) {
in_degree++;
}
if(G.Edge[no][i] == 1) {
out_degree++;
}
}
cout << "The in-degree of " << node << " is " << in_degree << endl;
cout << "The out-degree of " << node << " is " << out_degree << endl;
return 0;
}
阅读全文
相关推荐
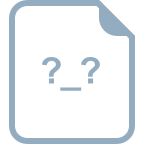
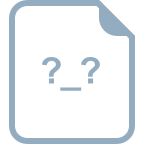
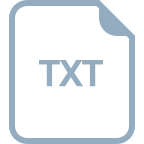
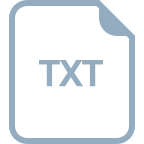
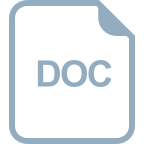
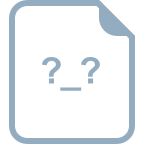
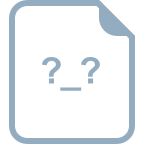
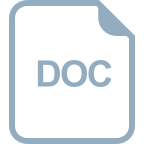
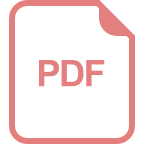
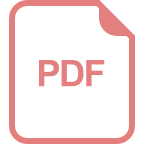
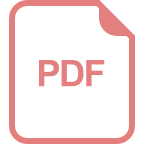
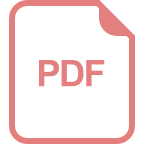
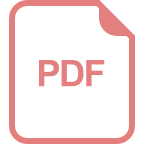
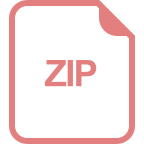
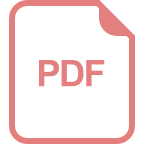
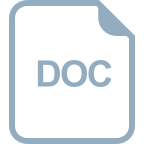
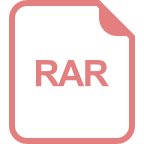