flink中如何把timestamp转成string
时间: 2024-05-02 17:19:49 浏览: 146
在 Flink 中,可以通过使用 TimestampAssigner 和 TimestampExtractor 接口来获取或设置时间戳,然后将时间戳转换为字符串。例如:
```
import org.apache.flink.api.common.functions.MapFunction;
import org.apache.flink.streaming.api.datastream.DataStream;
import org.apache.flink.streaming.api.environment.StreamExecutionEnvironment;
import org.apache.flink.streaming.api.functions.TimestampAssigner;
import org.apache.flink.streaming.api.functions.timestamps.BoundedOutOfOrdernessTimestampExtractor;
public class FlinkTimestampToStringExample {
public static void main(String[] args) throws Exception {
// set up the streaming execution environment
final StreamExecutionEnvironment env = StreamExecutionEnvironment.getExecutionEnvironment();
// create a data stream
DataStream<String> dataStream = env.fromElements(
"{\"event_time\": 1619023268, \"value\": 10}",
"{\"event_time\": 1619023271, \"value\": 20}",
"{\"event_time\": 1619023273, \"value\": 30}"
);
// extract the timestamp from the input data and assign it to each record
BoundedOutOfOrdernessTimestampExtractor<String> timestampExtractor = new BoundedOutOfOrdernessTimestampExtractor<String>(Time.seconds(10)) {
@Override
public long extractTimestamp(String record) {
JsonObject jsonObj = new JsonParser().parse(record).getAsJsonObject();
long eventTime = jsonObj.get("event_time").getAsLong();
return eventTime * 1000L; // convert seconds to milliseconds
}
};
// map the timestamp to a string representation
DataStream<String> resultStream = dataStream
.assignTimestampsAndWatermarks(timestampExtractor)
.map(new MapFunction<String, String>() {
@Override
public String map(String record) throws Exception {
JsonObject jsonObj = new JsonParser().parse(record).getAsJsonObject();
long eventTime = jsonObj.get("event_time").getAsLong();
String eventTimeString = Instant.ofEpochSecond(eventTime).toString(); // convert timestamp to ISO 8601 string
return "{\"event_time\": \"" + eventTimeString + "\", \"value\": " + jsonObj.get("value").getAsInt() + "}";
}
});
// print the output
resultStream.print();
// execute the program
env.execute("Flink Timestamp to String Example");
}
}
```
在以上代码中,我们首先将包含事件时间戳和数值的 JSON 数据流读入到 Flink 中。接下来,我们使用 BoundedOutOfOrdernessTimestampExtractor,从输入的 JSON 数据中提取出事件时间戳,并在每个记录上分配一个时间戳。时间戳分配程序还需要设置一个水位线延迟,以确保在乱序数据到达时能够正确地处理它们。
然后,我们使用一个 MapFunction 将每个记录中的时间戳转换为字符串格式。我们使用 Java 8 中的 java.time.Instant 类,将时间戳转换为符合 ISO 8601 标准的字符串表示形式。最后,我们将转换后的数据流打印出来。
阅读全文
相关推荐
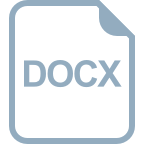
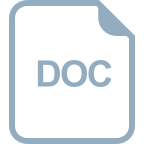
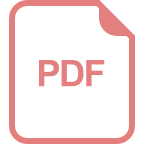


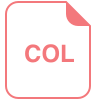
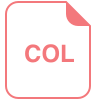
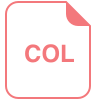
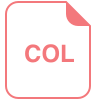
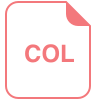
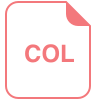








