python单目测距代码
时间: 2023-10-30 22:01:15 浏览: 32
单目测距一般使用的是立体视觉技术,需要使用双目摄像头或者深度摄像头进行拍摄。如果只有单个摄像头,需要通过一些算法对图像进行处理,提取出深度信息。以下是一个使用Python和OpenCV库实现的简单单目测距代码:
```python
import cv2
import numpy as np
# 读取图片
img = cv2.imread('image.jpg')
# 转为灰度图
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
# 使用高斯滤波平滑图像
gray = cv2.GaussianBlur(gray, (5, 5), 0)
# 边缘检测
edges = cv2.Canny(gray, 50, 150)
# 霍夫变换检测直线
lines = cv2.HoughLines(edges, 1, np.pi / 180, 200)
# 计算直线与图像边缘的交点
points = []
for line in lines:
rho, theta = line[0]
a = np.cos(theta)
b = np.sin(theta)
x0 = a * rho
y0 = b * rho
x1 = int(x0 + 1000 * (-b))
y1 = int(y0 + 1000 * a)
x2 = int(x0 - 1000 * (-b))
y2 = int(y0 - 1000 * a)
if x1 != x2:
k = (y2 - y1) / (x2 - x1)
b = y1 - k * x1
point = (int(-b/k), 0)
if point[0] > 0 and point[0] < img.shape[1]:
points.append(point)
# 计算距离
focal_length = 1000 # 焦距
real_width = 50 # 物体实际宽度
pixel_width = abs(points[0][0] - points[-1][0]) # 物体像素宽度
distance = focal_length * real_width / pixel_width
# 显示图像和结果
cv2.line(img, points[0], points[-1], (0, 0, 255), 2)
cv2.putText(img, '{:.2f}cm'.format(distance), (50, 50), cv2.FONT_HERSHEY_SIMPLEX, 1, (0, 255, 0), 2)
cv2.imshow('image', img)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
这段代码首先读取一张图片,然后将其转为灰度图,并使用高斯滤波平滑图像。接着使用边缘检测算法提取出图像的边缘,并使用霍夫变换检测出直线。然后通过计算直线与图像边缘的交点,得到物体的像素宽度。最后通过焦距等参数计算出物体的距离,并在图像上显示出距离信息和检测出的直线。
阅读全文
相关推荐
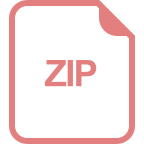



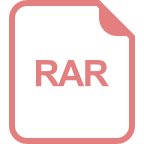
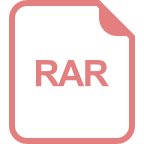
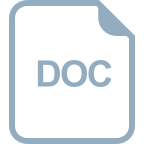







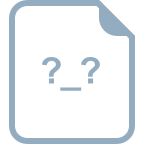
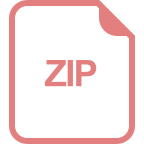