用lavarel写一段购物车功能
时间: 2024-02-24 17:56:46 浏览: 199
好的,这里是一个简单的 Laravel 购物车功能示例:
1. 创建一个名为 Cart 的类,用于管理购物车中的商品信息和操作,如添加、删除、获取商品列表、获取总价等。
```php
class Cart {
protected $items = []; // 购物车商品列表
// 添加商品到购物车
public function add($product_id, $product_name, $product_price, $quantity) {
if (isset($this->items[$product_id])) {
// 如果购物车中已经有该商品,则增加数量
$this->items[$product_id]['quantity'] += $quantity;
} else {
// 如果购物车中没有该商品,则添加新的商品信息
$item = [
'product_id' => $product_id,
'product_name' => $product_name,
'product_price' => $product_price,
'quantity' => $quantity
];
$this->items[$product_id] = $item;
}
}
// 从购物车中删除商品
public function remove($product_id) {
if (isset($this->items[$product_id])) {
unset($this->items[$product_id]);
}
}
// 获取购物车中的商品列表
public function getItems() {
return $this->items;
}
// 获取购物车中的商品总价
public function getTotal() {
$total = 0;
foreach ($this->items as $item) {
$total += $item['product_price'] * $item['quantity'];
}
return $total;
}
// 清空购物车
public function clear() {
$this->items = [];
}
}
```
2. 在 Laravel 中使用 Session 来存储购物车信息,使得购物车信息可以跨请求共享。在控制器中,可以通过以下代码来添加、删除、获取商品列表和获取总价等操作:
```php
class CartController extends Controller {
public function index() {
// 获取购物车信息
$cart = session('cart');
return view('cart.index', compact('cart'));
}
public function add($product_id, $product_name, $product_price, $quantity) {
// 添加商品到购物车
$cart = new Cart();
$cart->add($product_id, $product_name, $product_price, $quantity);
session(['cart' => $cart]);
return redirect()->back();
}
public function remove($product_id) {
// 从购物车中删除商品
$cart = session('cart');
$cart->remove($product_id);
session(['cart' => $cart]);
return redirect()->back();
}
public function clear() {
// 清空购物车
session(['cart' => null]);
return redirect()->back();
}
}
```
这是一个简单的购物车功能示例,你可以根据自己的需求来进行扩展和优化。
阅读全文
相关推荐
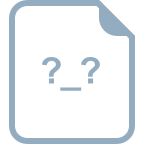
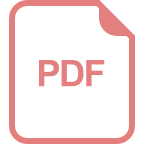
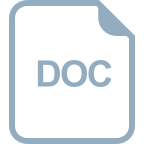
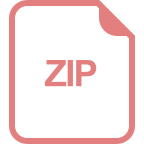
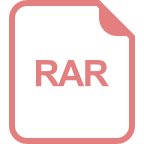
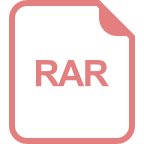
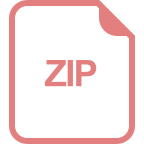
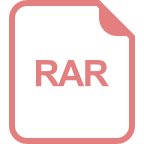
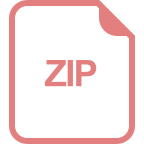
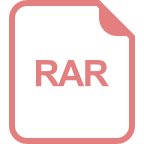
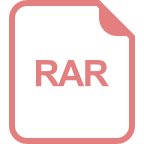
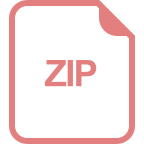
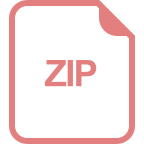