vue+openLayers 实现图层控制控件
时间: 2023-12-09 13:03:10 浏览: 180
要在 Vue 中使用 OpenLayers 实现图层控制控件,可以按照以下步骤进行操作:
1. 安装 OpenLayers 和 Vue:
```
npm install ol vue
```
2. 在 Vue 中引入 OpenLayers:
```javascript
import ol from 'ol'
import 'ol/ol.css'
```
3. 创建地图容器:
```html
<template>
<div ref="map" class="map"></div>
</template>
```
4. 在 Vue 的 mounted 钩子函数中创建地图:
```javascript
mounted() {
// 创建地图容器
const map = new ol.Map({
target: this.$refs.map,
layers: [
new ol.layer.Tile({
source: new ol.source.OSM()
})
],
view: new ol.View({
center: ol.proj.fromLonLat([116.3975, 39.9085]),
zoom: 12
})
});
this.map = map;
}
```
5. 创建图层控制控件:
```html
<template>
<div ref="map" class="map">
<div class="ol-control ol-custom-control">
<div class="ol-custom-control-header">图层控制</div>
<div class="ol-custom-control-body">
<div v-for="(layer, index) in layers" :key="index">
<input type="checkbox" :id="layer.name" v-model="layer.visible">
<label :for="layer.name">{{ layer.name }}</label>
</div>
</div>
</div>
</div>
</template>
```
6. 在 Vue 的 data 中定义图层数据和控件的状态:
```javascript
data() {
return {
map: null,
layers: [
{ name: 'OSM', visible: true, layer: new ol.layer.Tile({ source: new ol.source.OSM() }) },
{ name: 'Bing Maps', visible: false, layer: new ol.layer.Tile({ source: new ol.source.BingMaps({ key: 'your-bingmaps-api-key', imagerySet: 'Road' }) }) }
]
}
}
```
7. 在 Vue 的 watch 中监听图层状态的变化并更新地图:
```javascript
watch: {
layers: {
deep: true,
handler(layers) {
const map = this.map;
map.getLayers().clear();
layers.forEach(layer => {
if (layer.visible) {
map.addLayer(layer.layer);
}
});
}
}
}
```
通过以上步骤就可以在 Vue 中实现图层控制控件了。需要注意的是,在实际应用中,可以根据需要自定义控件的样式和布局。
阅读全文
相关推荐
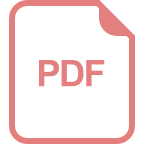
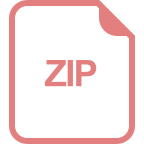
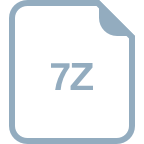















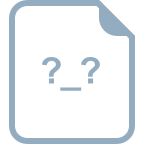