使用Java unit test测试下面代码public int onStartCommand(Intent intent, int flags, int startId) { if (intent != null) { String action = intent.getAction(); LogUtils.logI(TAG, "onStartCommand: action " + action); if (Config.ACTION_START_MUSIC.equals(action)) { boolean fromRecovery = intent.getBooleanExtra(Config.KEY_FROM_RECOVERY, false); Log.i(TAG, "onStartCommand: fromRecovery " + fromRecovery); if (fromRecovery) { mHasShowUsbTab = false; checkBootAnim(); } } else if (Config.ACTION_MUSIC_CMD.equals(action)) { mPlayer.change(); } else if ("com.music.action.play_first".equals(action)) { mPlayer.play(0, PlayParam.FROM_UNDEFINE); } else if (Config.ACTION_CLOSE_VIDEO.equals(action)) { EventBus.getDefault().post(new Event.Finish()); } } return START_STICKY; }
时间: 2024-02-14 19:10:30 浏览: 112
可以使用JUnit框架编写测试用例,测试onStartCommand()方法的不同分支。具体步骤如下:
1. 创建一个测试类,导入需要的包:
```java
import org.junit.Test;
import org.junit.runner.RunWith;
import org.mockito.Mock;
import org.mockito.junit.MockitoJUnitRunner;
import android.content.Intent;
import static org.mockito.Mockito.*;
@RunWith(MockitoJUnitRunner.class)
public class MyServiceTest {
@Mock
private MyService mService;
@Mock
private Intent mMockIntent;
@Test
public void testOnStartCommand_ActionStartMusic_FromRecovery() {
// 设置Intent的Action和参数
when(mMockIntent.getAction()).thenReturn(Config.ACTION_START_MUSIC);
when(mMockIntent.getBooleanExtra(Config.KEY_FROM_RECOVERY, false)).thenReturn(true);
// 调用onStartCommand()方法
mService.onStartCommand(mMockIntent, 0, 0);
// 验证相关的方法是否被调用
verify(mService).checkBootAnim();
}
@Test
public void testOnStartCommand_ActionStartMusic_NotFromRecovery() {
// 设置Intent的Action和参数
when(mMockIntent.getAction()).thenReturn(Config.ACTION_START_MUSIC);
when(mMockIntent.getBooleanExtra(Config.KEY_FROM_RECOVERY, false)).thenReturn(false);
// 调用onStartCommand()方法
mService.onStartCommand(mMockIntent, 0, 0);
// 验证相关的方法是否被调用
verify(mService, never()).checkBootAnim();
}
// TODO: 添加其他测试用例
}
```
2. 在测试方法中,设置模拟的Intent对象的Action和参数,并调用onStartCommand()方法。
3. 验证onStartCommand()方法中涉及到的其他方法是否被正确地调用。
4. 运行测试用例,查看测试结果是否符合预期。
需要注意的是,由于onStartCommand()方法中涉及到了一些外部依赖(如LogUtils、mPlayer、EventBus等),因此在测试中需要使用Mockito等框架来模拟这些依赖,以确保测试的独立性和可重复性。
阅读全文
相关推荐
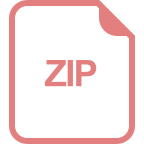
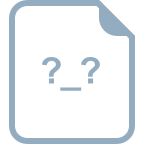
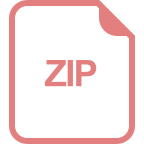
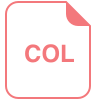
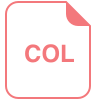
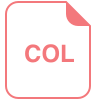









