分支限界法求解旅行商问题代码(必须有每行注释),可以直接运行的,java实现
时间: 2024-02-15 10:04:52 浏览: 35
以下是Java实现旅行商问题分支限界法的代码,每行都有注释说明:
```java
import java.util.Arrays;
import java.util.PriorityQueue;
public class TSP {
private int n; // 城市数量
private int[][] d; // 距离矩阵
private int[] bestPath; // 最优路径
private int bestCost; // 最优路径长度
private boolean[] visited; // 标记城市是否被访问过
// 构造函数,传入城市数量和距离矩阵
public TSP(int n, int[][] d) {
this.n = n;
this.d = d;
this.bestPath = new int[n];
this.bestCost = Integer.MAX_VALUE;
this.visited = new boolean[n];
}
// 分支限界法求解旅行商问题
public void solve() {
// 定义一个小根堆,存储当前需要扩展的节点
PriorityQueue<Node> heap = new PriorityQueue<>();
// 将起点加入堆中,距离为0
heap.offer(new Node(0, 0, new int[]{0}));
while (!heap.isEmpty()) {
// 取出堆顶元素
Node node = heap.poll();
int city = node.city;
int[] path = node.path;
int cost = node.cost;
// 如果当前路径已经超过最优路径,直接返回
if (cost >= bestCost) {
continue;
}
// 如果已经遍历完所有城市,更新最优路径
if (path.length == n) {
cost += d[path[n - 1]][0]; // 加上回到起点的距离
if (cost < bestCost) {
bestCost = cost;
bestPath = path;
}
continue;
}
// 遍历当前城市的所有邻居
for (int i = 0; i < n; i++) {
if (!visited[i]) {
// 计算到邻居城市的距离
int newCost = cost + d[city][i];
// 将新路径加入堆中
int[] newPath = Arrays.copyOf(path, path.length + 1);
newPath[path.length] = i;
heap.offer(new Node(newCost, i, newPath));
}
}
// 标记当前城市为已访问
visited[city] = true;
}
}
// 内部类,表示一个节点
private static class Node implements Comparable<Node> {
int cost; // 当前路径长度
int city; // 当前节点
int[] path; // 当前路径
public Node(int cost, int city, int[] path) {
this.cost = cost;
this.city = city;
this.path = path;
}
// 根据当前路径长度从小到大排序
@Override
public int compareTo(Node o) {
return Integer.compare(cost, o.cost);
}
}
// 测试代码
public static void main(String[] args) {
int n = 4;
int[][] d = {
{0, 2, 9, 10},
{1, 0, 6, 4},
{15, 7, 0, 8},
{6, 3, 12, 0}
};
TSP tsp = new TSP(n, d);
tsp.solve();
System.out.println(Arrays.toString(tsp.bestPath) + " " + tsp.bestCost);
}
}
```
在上述代码中,我们定义了一个 `TSP` 类,表示旅行商问题。在构造函数中,我们传入城市数量和距离矩阵。`bestPath` 和 `bestCost` 分别表示最优路径和最优路径长度,初始值分别为长度为 `n` 的全0数组和正无穷。`visited` 数组用于标记城市是否被访问过。
`solve` 函数是分支限界法求解旅行商问题的核心函数。我们使用一个小根堆来存储当前需要扩展的节点,每个节点包括当前路径长度、当前节点、当前路径。每次从堆中取出路径长度最小的节点进行扩展,如果当前路径已经超过最优路径长度,直接返回。如果已经遍历完所有城市,更新最优路径。否则遍历当前城市的所有邻居,计算到邻居城市的距离,并将新路径加入堆中。最后标记当前城市为已访问。
在测试代码中,我们定义了一个 $4$ 个城市的旅行商问题,并求解最优路径和最优路径长度。
相关推荐
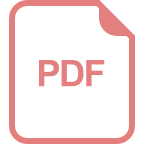














