帮我用springboot+mybatis+vue写一份汽车信息管理系统
时间: 2023-07-29 07:07:40 浏览: 59
好的,我可以为您提供一个使用Spring Boot、MyBatis和Vue.js的汽车信息管理系统示例。
首先,我们需要创建一个Spring Boot项目,并且添加以下依赖项:
```xml
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.mybatis.spring.boot</groupId>
<artifactId>mybatis-spring-boot-starter</artifactId>
<version>2.2.0</version>
</dependency>
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
</dependency>
<dependency>
<groupId>com.github.pagehelper</groupId>
<artifactId>pagehelper</artifactId>
<version>5.1.8</version>
</dependency>
</dependencies>
```
然后,我们需要创建一个实体类来表示汽车:
```java
public class Car {
private Long id;
private String make;
private String model;
private Integer year;
// getters and setters
}
```
接下来,我们需要创建一个Mapper来处理汽车实体的CRUD操作:
```java
@Mapper
public interface CarMapper {
@Select("SELECT * FROM cars")
List<Car> getAllCars();
@Select("SELECT * FROM cars WHERE id = #{id}")
Car getCarById(Long id);
@Insert("INSERT INTO cars(make, model, year) VALUES(#{make}, #{model}, #{year})")
@Options(useGeneratedKeys = true, keyProperty = "id", keyColumn = "id")
void createCar(Car car);
@Update("UPDATE cars SET make = #{make}, model = #{model}, year = #{year} WHERE id = #{id}")
void updateCar(Car car);
@Delete("DELETE FROM cars WHERE id = #{id}")
void deleteCar(Long id);
}
```
然后,我们需要创建一个控制器来处理HTTP请求:
```java
@RestController
@RequestMapping("/cars")
public class CarController {
@Autowired
private CarMapper carMapper;
@GetMapping("/")
public List<Car> getAllCars() {
return carMapper.getAllCars();
}
@PostMapping("/")
public void createCar(@RequestBody Car car) {
carMapper.createCar(car);
}
@GetMapping("/{id}")
public Car getCarById(@PathVariable Long id) {
return carMapper.getCarById(id);
}
@PutMapping("/{id}")
public void updateCar(@PathVariable Long id, @RequestBody Car car) {
car.setId(id);
carMapper.updateCar(car);
}
@DeleteMapping("/{id}")
public void deleteCar(@PathVariable Long id) {
carMapper.deleteCar(id);
}
}
```
最后,我们需要使用Vue.js创建前端界面。这里只提供一个简单的示例:
```vue
<template>
<div>
<h2>Cars</h2>
<table>
<thead>
<tr>
<th>ID</th>
<th>Make</th>
<th>Model</th>
<th>Year</th>
<th></th>
</tr>
</thead>
<tbody>
<tr v-for="car in cars" :key="car.id">
<td>{{ car.id }}</td>
<td>{{ car.make }}</td>
<td>{{ car.model }}</td>
<td>{{ car.year }}</td>
<td>
<button @click="editCar(car)">Edit</button>
<button @click="deleteCar(car.id)">Delete</button>
</td>
</tr>
</tbody>
</table>
<h2 v-if="selectedCar">Edit Car</h2>
<form v-if="selectedCar" @submit.prevent="saveCar">
<label>Make:</label>
<input type="text" v-model="selectedCar.make" required>
<br>
<label>Model:</label>
<input type="text" v-model="selectedCar.model" required>
<br>
<label>Year:</label>
<input type="number" v-model="selectedCar.year" required>
<br>
<button type="submit">Save</button>
<button type="button" @click="cancelEdit">Cancel</button>
</form>
<hr>
<h2>Create Car</h2>
<form @submit.prevent="createCar">
<label>Make:</label>
<input type="text" v-model="newCar.make" required>
<br>
<label>Model:</label>
<input type="text" v-model="newCar.model" required>
<br>
<label>Year:</label>
<input type="number" v-model="newCar.year" required>
<br>
<button type="submit">Create</button>
</form>
</div>
</template>
<script>
export default {
data() {
return {
cars: [],
newCar: {
make: "",
model: "",
year: null,
},
selectedCar: null,
};
},
created() {
this.fetchCars();
},
methods: {
fetchCars() {
fetch("/cars/")
.then((response) => response.json())
.then((data) => (this.cars = data));
},
createCar() {
fetch("/cars/", {
method: "POST",
headers: {
"Content-Type": "application/json",
},
body: JSON.stringify(this.newCar),
})
.then(() => {
this.fetchCars();
this.newCar.make = "";
this.newCar.model = "";
this.newCar.year = null;
})
.catch((error) => console.error(error));
},
editCar(car) {
this.selectedCar = Object.assign({}, car);
},
saveCar() {
fetch(`/cars/${this.selectedCar.id}`, {
method: "PUT",
headers: {
"Content-Type": "application/json",
},
body: JSON.stringify(this.selectedCar),
})
.then(() => {
this.fetchCars();
this.cancelEdit();
})
.catch((error) => console.error(error));
},
cancelEdit() {
this.selectedCar = null;
},
deleteCar(id) {
fetch(`/cars/${id}`, {
method: "DELETE",
})
.then(() => this.fetchCars())
.catch((error) => console.error(error));
},
},
};
</script>
```
现在,我们已经完成了汽车信息管理系统的开发。您可以使用浏览器访问该应用程序,并测试它。
相关推荐
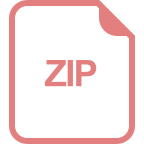
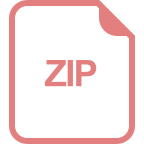
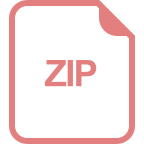
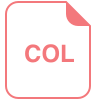
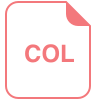
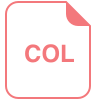
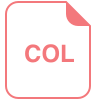
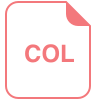







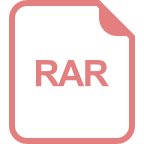
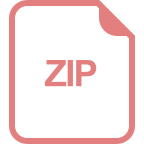
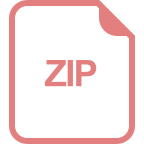
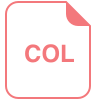