c语言反转单链表
时间: 2023-08-24 17:16:05 浏览: 90
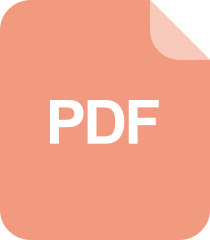
C语言实现单链表反转
反转单链表的基本思路是使用三个指针 `pre, cur, next`,将当前节点 `cur` 的指针指向前一个节点 `pre`,然后依次更新 `pre, cur, next` 指针,直到 `cur` 走到链表的末尾。反转完成后,将链表的头节点指向原链表的尾节点即可。
下面是用 C 语言实现反转单链表的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
typedef struct ListNode {
int val;
struct ListNode *next;
} ListNode;
ListNode* reverseList(ListNode* head) {
ListNode *pre = NULL;
ListNode *cur = head;
ListNode *next;
while (cur) {
next = cur->next;
cur->next = pre;
pre = cur;
cur = next;
}
return pre;
}
int main() {
ListNode *head = (ListNode*)malloc(sizeof(ListNode));
head->val = 1;
head->next = (ListNode*)malloc(sizeof(ListNode));
head->next->val = 2;
head->next->next = (ListNode*)malloc(sizeof(ListNode));
head->next->next->val = 3;
head->next->next->next = NULL;
ListNode *new_head = reverseList(head);
while (new_head) {
printf("%d ", new_head->val);
new_head = new_head->next;
}
return 0;
}
```
在上面的代码中,我们创建了一个包含三个节点的链表,然后调用 `reverseList` 函数对链表进行反转,最后输出反转后的链表。
阅读全文
相关推荐
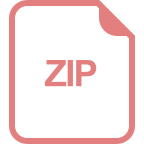
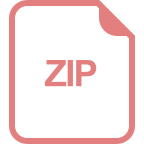
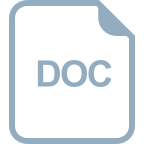
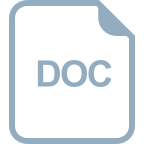
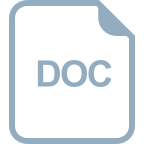
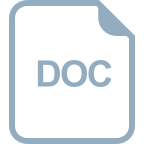
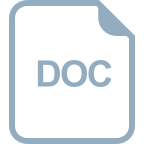



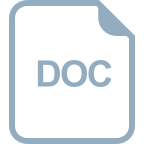
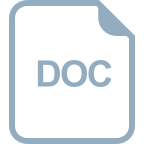
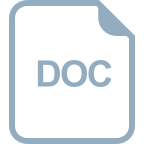
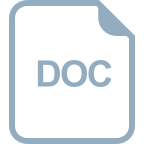
