用C语言实现单链表递归反转,并附带解释
时间: 2023-06-03 22:04:02 浏览: 146
好的,可以开始讨论如何用C语言实现单链表递归反转。
首先,我们需要了解单链表和递归的概念。单链表是由一系列节点组成的数据结构,每个节点都包含一个数据元素和一个指向下一个节点的指针。递归则是一种通过函数自身不断调用来解决问题的方法。
在单链表中,反转的关键在于修改每个节点的指针指向前一个节点。而递归则可以通过不断递归到链表尾部,并在回溯的过程中修改指针来完成反转。
下面是用C语言实现单链表递归反转的代码:
```
#include <stdio.h>
#include <stdlib.h>
struct Node {
int data;
struct Node* next;
};
struct Node* reverse(struct Node* head) {
if (head == NULL || head->next == NULL) {
return head;
}
struct Node* newHead = reverse(head->next);
head->next->next = head;
head->next = NULL;
return newHead;
}
int main() {
struct Node* head = NULL;
// 创建链表
head = (struct Node*)malloc(sizeof(struct Node));
head->data = 1;
head->next = (struct Node*)malloc(sizeof(struct Node));
head->next->data = 2;
head->next->next = (struct Node*)malloc(sizeof(struct Node));
head->next->next->data = 3;
head->next->next->next = (struct Node*)malloc(sizeof(struct Node));
head->next->next->next->data = 4;
head->next->next->next->next = NULL;
// 反转链表
head = reverse(head);
// 打印链表
while (head != NULL) {
printf("%d ", head->data);
head = head->next;
}
return 0;
}
```
在上面的代码中,我们定义了一个Node结构体来表示节点,其中包括了数据和指向下一个节点的指针。reverse函数通过递归来实现链表反转,并返回反转后的新头节点。在递归的过程中,我们先递归到链表尾部,然后在回溯的过程中修改每个节点的指针,实现链表反转。
希望这个例子能帮助您更好地理解单链表递归反转的原理。
阅读全文
相关推荐
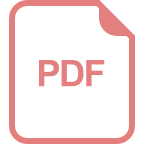

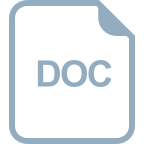
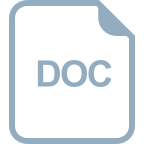
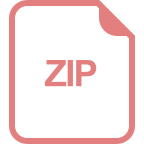
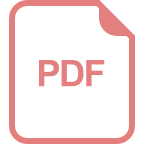
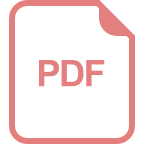
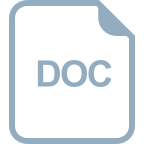
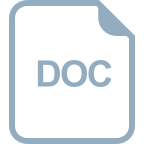
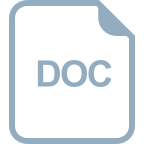
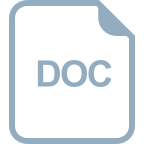
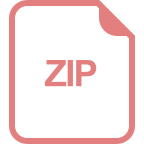
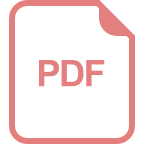
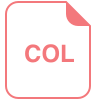
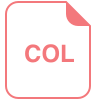

