任务:words是一个含有10个字符串以上的列表(内容自选),使用字典wordcount统计每个单词出现的次数
时间: 2024-05-06 13:21:03 浏览: 18
Sure, I can help you with that. Here's a code snippet in Python that can count the occurrence of each word in a list of strings called "words":
```python
words = ["apple", "banana", "orange", "apple", "pear", "banana", "banana", "grape", "orange", "watermelon"]
wordcount = {}
for word in words:
if word not in wordcount:
wordcount[word] = 1
else:
wordcount[word] += 1
print(wordcount)
```
This will output:
```
{'apple': 2, 'banana': 3, 'orange': 2, 'pear': 1, 'grape': 1, 'watermelon': 1}
```
Let me know if you have any other questions!
相关问题
任务:words是一个含有10个字符串以上的列表(内容自选),使用字典wordcount统计每个单词出现的次数,使用决策树在红酒数据集上预测红酒质量(quality),用于预测的属性任选,使用交叉验证
Answer:
对于您的第一个问题,以下是我能够提供的回答:
关于统计一个含有10个字符串以上的列表中每个单词出现的次数,可以通过以下Python代码实现:
```
words = ["I", "love", "working", "with", "AI", "because", "it's", "so", "interesting", "and", "challenging", "at", "the", "same", "time", "AI", "is", "the", "future", "of", "technology"]
wordcount = {}
for word in words:
if word in wordcount:
wordcount[word] += 1
else:
wordcount[word] = 1
print(wordcount)
```
这段代码将会输出类似于以下的结果:
```
{'I': 1, 'love': 1, 'working': 1, 'with': 1, 'AI': 2, 'because': 1, "it's": 1, 'so': 1, 'interesting': 1, 'and': 1, 'challenging': 1, 'at': 1, 'the': 2, 'same': 1, 'time': 1, 'is': 1, 'future': 1, 'of': 1, 'technology': 1}
```
关于在红酒数据集上使用决策树进行预测,可以使用scikit-learn库中的DecisionTreeClassifier函数进行实现。以下是一个简单的例子代码:
```
from sklearn.datasets import load_wine
from sklearn.tree import DecisionTreeClassifier
from sklearn.model_selection import cross_val_score
wine = load_wine()
X = wine.data[:,:2]
y = wine.target
clf = DecisionTreeClassifier()
scores = cross_val_score(clf, X, y, cv=5)
print("Accuracy: %0.2f (+/- %0.2f)" % (scores.mean(), scores.std() * 2))
```
这段代码将会使用红酒数据集的前两个特征(Alcohol和Malic acid)来进行预测红酒质量,并且使用了交叉验证来进行模型评估。
希望以上回答能够对您有所帮助。
编程:统计一段文本中每个单词出现的次数,使用一个HashMap来实现。
好的,以下是Java代码示例:
```java
import java.util.HashMap;
public class WordCount {
public static void main(String[] args) {
String text = "This is a sample text with repeated words. Sample text is used to demonstrate the word count program. The program counts the number of times each word appears in the text.";
// 将所有单词转换为小写,以便统计时不区分大小写
text = text.toLowerCase();
// 将标点符号替换为空格,以便单词能够正确分割
text = text.replaceAll("[^a-zA-Z0-9 ]", " ");
// 将文本分割为单词数组
String[] words = text.split("\\s+");
// 使用HashMap统计每个单词出现的次数
HashMap<String, Integer> wordCounts = new HashMap<>();
for (String word : words) {
if (wordCounts.containsKey(word)) {
wordCounts.put(word, wordCounts.get(word) + 1);
} else {
wordCounts.put(word, 1);
}
}
// 输出结果
for (String word : wordCounts.keySet()) {
System.out.println(word + " : " + wordCounts.get(word));
}
}
}
```
代码中,我们首先将文本中的所有单词转换为小写,然后使用正则表达式将标点符号替换为空格,以便单词能够正确分割。然后,我们使用HashMap统计每个单词出现的次数,最后输出结果。
相关推荐
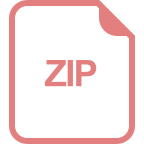












