在Linux系统中使用makefile建立一个通讯录哈希表程序,联系人与电话号码一一对应,实现联系人输入、联系人排序,联系人删除、联系人查找,并封装函数
时间: 2024-05-14 21:19:37 浏览: 171
好的,我明白了。关于你的问题,我可以为你提供思路和部分代码实现。
思路:
1. 首先,你需要设计一个哈希表的结构体,包括联系人和电话号码的信息,以及哈希表的大小和当前元素数量等信息。
2. 然后,你需要实现添加联系人和电话号码的函数,以及根据联系人进行删除和查找的函数。
3. 接下来,你需要实现对联系人的排序,可以采用冒泡排序等简单排序算法。
4. 最后,你需要封装这些函数,让它们成为可调用的接口。
代码实现:
下面是一个简单的 makefile 代码,用于编译哈希表程序:
```
CC = gcc
CFLAGS = -Wall -g
all: contact
contact: contact.o hash.o
$(CC) $(CFLAGS) -o $@ $^
contact.o: contact.c hash.h
$(CC) $(CFLAGS) -c $<
hash.o: hash.c hash.h
$(CC) $(CFLAGS) -c $<
clean:
rm -f *.o contact
```
这是哈希表的结构体定义:
```
#define SIZE 1000
typedef struct {
char name[20];
char phone[20];
} Contact;
typedef struct {
Contact entries[SIZE];
int size;
} HashTable;
```
下面是添加、删除和查找联系人的函数:
```
void add_contact(HashTable *table, char *name, char *phone)
{
int index = hash(name);
strcpy(table->entries[index].name, name);
strcpy(table->entries[index].phone, phone);
table->size++;
}
void remove_contact(HashTable *table, char *name)
{
int index = hash(name);
if (strcmp(table->entries[index].name, name) == 0) {
table->size--;
table->entries[index] = (Contact){{0}, {0}};
} else {
printf("Error: Contact not found.\n");
}
}
void find_contact(HashTable *table, char *name)
{
int index = hash(name);
if (strcmp(table->entries[index].name, name) == 0) {
printf("%s: %s\n", table->entries[index].name, table->entries[index].phone);
} else {
printf("Error: Contact not found.\n");
}
}
```
这是排序联系人的函数:
```
void sort_contacts(HashTable *table)
{
int i, j;
Contact temp;
for (i = 0; i < table->size - 1; i++) {
for (j = 0; j < table->size - i - 1; j++) {
if (strcmp(table->entries[j].name, table->entries[j+1].name) > 0) {
temp = table->entries[j];
table->entries[j] = table->entries[j+1];
table->entries[j+1] = temp;
}
}
}
}
```
最后,这是封装函数的示例:
```
void add_contact_wrapper(char *name, char *phone)
{
add_contact(&table, name, phone);
}
void remove_contact_wrapper(char *name)
{
remove_contact(&table, name);
}
void find_contact_wrapper(char *name)
{
find_contact(&table, name);
}
void sort_contacts_wrapper()
{
sort_contacts(&table);
}
```
注意,这只是一个简单的示例,可能存在一些错误和不完善之处。如果你有更具体的要求和问题,可以再与我进行对话。
阅读全文
相关推荐





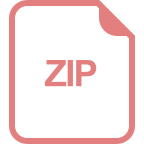






