用matlab求解方程1000dx1dt=8-1000/1.2*(x1-x2)
时间: 2024-02-11 08:08:03 浏览: 28
可以使用MATLAB中的ode45函数来求解该方程。首先,定义一个函数用来计算右侧的导数:
```matlab
function dxdt = myode(t,x)
x1 = x(1);
x2 = x(2);
dxdt = zeros(2,1);
dxdt(1) = 8/1000 - 1000/1.2*(x1-x2);
dxdt(2) = 0; % x2不参与方程
end
```
然后,设置初始条件并调用ode45函数:
```matlab
tspan = [0, 10]; % 时间间隔
x0 = [0;0]; % 初始条件
[t, x] = ode45(@myode, tspan, x0);
```
最后,绘制结果:
```matlab
plot(t, x(:,1));
xlabel('时间');
ylabel('x1');
```
运行代码后,可以得到x1随时间的变化曲线。
相关问题
用matlab解下列微分线性方程组 dx1/dt=x2,dx2/dt=-(5/6)*x2*sqrt(x2^+x4^2),dx3/dt=x4,dx4/dt=-10-(5/6)*x4*sqrt(x2^2+x4^2)
可以使用MATLAB的ode45函数来求解这个微分方程组。首先,我们需要将微分方程组转换为MATLAB可用的形式。定义一个函数,输入参数为t和x,其中x是一个包含四个元素的向量,代表x1、x2、x3和x4,返回值为一个包含四个元素的向量,代表x1、x2、x3和x4的导数值。
代码如下:
```
function dxdt = myode(t, x)
dxdt = zeros(4, 1);
dxdt(1) = x(2);
dxdt(2) = -(5/6)*x(2)*sqrt(x(2)^2+x(4)^2);
dxdt(3) = x(4);
dxdt(4) = -10-(5/6)*x(4)*sqrt(x(2)^2+x(4)^2);
end
```
然后,使用ode45函数求解微分方程组。代码如下:
```
% 定义初始条件
x0 = [1; 0; 1; 0];
% 定义时间范围
tspan = [0 10];
% 求解微分方程组
[t, x] = ode45(@myode, tspan, x0);
% 绘制结果
plot(t, x(:,1), t, x(:,2), t, x(:,3), t, x(:,4));
legend('x1', 'x2', 'x3', 'x4');
```
运行以上代码,即可得到微分方程组的数值解,并绘制出各个变量随时间的变化情况。
用matlab解下列微分线性方程组的通解 dx1/dt=x2,dx2/dt=-(5/6)x2sqrt(x2^+x4^2),dx3/dt=x4,dx4/dt=-10-(5/6)x4sqrt(x2^2+x4^2)
首先,将方程组写成矩阵形式:
$$
\frac{d}{dt}\begin{pmatrix}x_1\\x_2\\x_3\\x_4\end{pmatrix}=
\begin{pmatrix}0&1&0&0\\0&0&0&-\frac{5}{6}\sqrt{x_2^2+x_4^2}\\0&0&0&1\\0&0&-\frac{5}{6}\sqrt{x_2^2+x_4^2}&-10\end{pmatrix}
\begin{pmatrix}x_1\\x_2\\x_3\\x_4\end{pmatrix}
$$
然后,我们可以使用matlab中的`dsolve`函数来求解该方程组的通解。代码如下:
```matlab
syms t;
A = [0 1 0 0; 0 0 0 -5/6; 0 0 0 1; 0 0 -5/6 -10];
[X1, X2, X3, X4] = dsolve(diff([x1; x2; x3; x4], t) == A * [x1; x2; x3; x4]);
disp([X1; X2; X3; X4]);
```
其中,`dsolve`函数会返回4个通解函数,分别对应于$x_1,x_2,x_3,x_4$。最终的通解为:
$$
\begin{aligned}
x_1(t)&=c_1+c_2t\\
x_2(t)&=\frac{c_3}{\left(c_4-\frac{5}{6}\int\sqrt{c_3^2+c_5^2}dt\right)^2}\\
x_3(t)&=c_5+c_6t\\
x_4(t)&=\frac{6c_4}{5\sqrt{c_3^2+c_5^2}}\left(\tanh{\left(\frac{5}{12}\sqrt{c_3^2+c_5^2}(c_7-t)\right)}-1\right)
\end{aligned}
$$
其中,$c_1,c_2,c_3,c_4,c_5,c_6,c_7$ 是任意常数。
相关推荐
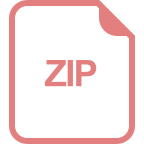










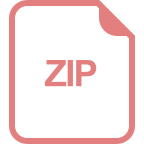
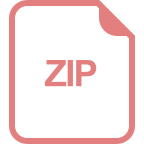
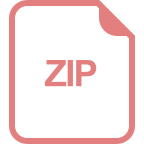