【问题描述】分别用字符数组或字符指针做函数参数,在字符串中删除与某字符相同的字符,执行两次删除和结果输出显示。若删除后为空串,则输出NULL。 void Delete1(char s[ ], char ch); void Delete2(char *p, char ch); 【样例输入】 abcdabcd c d 【样例输出】 abdabd abab
时间: 2023-11-27 20:53:29 浏览: 32
以下是代码实现:
```c++
#include <iostream>
#include <cstring>
using namespace std;
void Delete1(char s[], char ch) {
int len = strlen(s);
int i = 0, j = 0;
while (j < len) {
if (s[j] != ch) {
s[i++] = s[j];
}
j++;
}
s[i] = '\0';
}
void Delete2(char *p, char ch) {
int len = strlen(p);
int i = 0, j = 0;
while (j < len) {
if (*(p+j) != ch) {
*(p+i) = *(p+j);
i++;
}
j++;
}
*(p+i) = '\0';
}
int main() {
char s1[100], s2[100], ch1, ch2;
cout << "请输入字符串和要删除的字符(用空格隔开):";
cin >> s1 >> ch1;
strcpy(s2, s1); // 备份一份字符串
Delete1(s1, ch1);
Delete2(s2, ch1);
cout << "删除一次后的结果:" << s1 << endl;
cout << "删除两次后的结果:" << s2 << endl;
// 再进行一次删除操作
ch2 = s2[0];
strcpy(s1, s2);
Delete1(s1, ch2);
Delete2(s2, ch2);
if (strlen(s1) == 0) cout << "NULL" << endl;
else cout << "删除三次后的结果:" << s1 << endl;
if (strlen(s2) == 0) cout << "NULL" << endl;
else cout << "删除三次后的结果:" << s2 << endl;
return 0;
}
```
其中,`Delete1` 函数使用字符数组作为参数,`Delete2` 函数使用字符指针作为参数。在 `main` 函数中,先输入字符串和要删除的字符,然后分别调用两个函数进行删除操作,并输出删除一次和删除两次后的结果。接着再进行一次删除操作,并判断结果是否为空字符串,若为空则输出 "NULL"。最后输出删除三次后的结果。
相关推荐
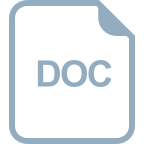
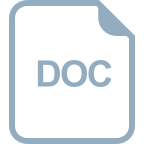
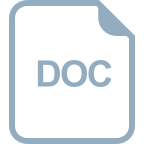
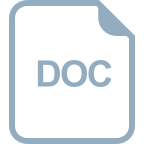
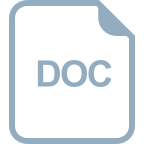
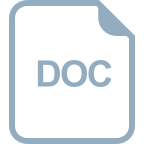
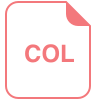
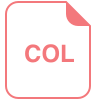
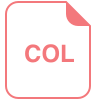
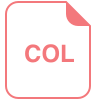
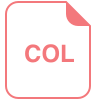




