javamap函数的使用
时间: 2024-04-04 16:12:28 浏览: 123
Java中的Map是一种常用的数据结构,它提供了一种将键映射到值的方式。Map中每个键最多只能映射到一个值,而且不能有重复的键。Java中的Map有多种实现方式,如HashMap、TreeMap等。
Map的put方法用于将键值对存储到Map中,get方法用于获取指定键对应的值。除此之外,Map还提供了一些其他的常用方法,如containsKey、containsValue、remove等。
下面是一个使用HashMap实现的Map的例子,展示了put、get、containsKey等方法的使用:
```
import java.util.HashMap;
import java.util.Map;
public class MapExample {
public static void main(String[] args) {
// 创建一个HashMap实例
Map<String, Integer> map = new HashMap<>();
// 添加键值对
map.put("apple", 1);
map.put("banana", 2);
map.put("orange", 3);
// 获取键对应的值
int value = map.get("banana");
System.out.println("The value of banana is " + value);
// 判断是否包含指定的键
boolean containsKey = map.containsKey("orange");
System.out.println("Does the map contain key 'orange'? " + containsKey);
// 判断是否包含指定的值
boolean containsValue = map.containsValue(4);
System.out.println("Does the map contain value 4? " + containsValue);
// 删除指定的键值对
map.remove("apple");
// 输出所有的键值对
for (Map.Entry<String, Integer> entry : map.entrySet()) {
System.out.println(entry.getKey() + " - " + entry.getValue());
}
}
}
```
运行上述代码,输出结果如下:
```
The value of banana is 2
Does the map contain key 'orange'? true
Does the map contain value 4? false
banana - 2
orange - 3
```
可以看到,Map提供了一系列方便的方法,可以方便地进行键值对的存储、获取、删除等操作。
阅读全文
相关推荐
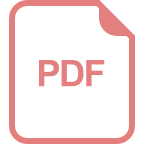
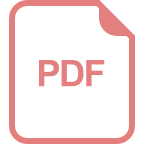
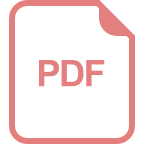














