java实现输入一个年份输出是在屏幕上显示该年的日历。假定输入的年份在1980-2050年之间;
时间: 2023-06-25 13:04:34 浏览: 65
以下是Java实现输入一个年份输出该年的日历的代码:
```java
import java.util.Scanner;
public class Calendar {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.print("请输入一个年份(1980-2050):");
int year = scanner.nextInt();
if (year < 1980 || year > 2050) {
System.out.println("输入的年份不在1980-2050年之间!");
return;
}
int weekDay = getWeekDay(year, 1, 1); // 获取该年1月1日是星期几
int daysOfMonth; // 当前月份的天数
for (int month = 1; month <= 12; month++) {
switch (month) {
case 1:
System.out.println("------------" + year + "年1月------------");
daysOfMonth = 31;
break;
case 2:
System.out.println("------------" + year + "年2月------------");
if (isLeapYear(year)) {
daysOfMonth = 29;
} else {
daysOfMonth = 28;
}
break;
case 3:
System.out.println("------------" + year + "年3月------------");
daysOfMonth = 31;
break;
case 4:
System.out.println("------------" + year + "年4月------------");
daysOfMonth = 30;
break;
case 5:
System.out.println("------------" + year + "年5月------------");
daysOfMonth = 31;
break;
case 6:
System.out.println("------------" + year + "年6月------------");
daysOfMonth = 30;
break;
case 7:
System.out.println("------------" + year + "年7月------------");
daysOfMonth = 31;
break;
case 8:
System.out.println("------------" + year + "年8月------------");
daysOfMonth = 31;
break;
case 9:
System.out.println("------------" + year + "年9月------------");
daysOfMonth = 30;
break;
case 10:
System.out.println("------------" + year + "年10月-----------");
daysOfMonth = 31;
break;
case 11:
System.out.println("------------" + year + "年11月-----------");
daysOfMonth = 30;
break;
default:
System.out.println("------------" + year + "年12月-----------");
daysOfMonth = 31;
}
// 输出日历表头
System.out.println("日\t一\t二\t三\t四\t五\t六");
// 输出该月第一天之前的空格
for (int i = 0; i < weekDay; i++) {
System.out.print("\t");
}
// 输出该月的每一天
for (int day = 1; day <= daysOfMonth; day++) {
System.out.print(day + "\t");
weekDay++;
if (weekDay == 7) { // 周六之后换行
System.out.println();
weekDay = 0;
}
}
System.out.println(); // 换行
}
}
// 判断是否闰年
public static boolean isLeapYear(int year) {
return (year % 4 == 0 && year % 100 != 0) || year % 400 == 0;
}
// 获取某一天是星期几,0表示星期日,1表示星期一,依次类推
public static int getWeekDay(int year, int month, int day) {
if (month == 1 || month == 2) { // 1月和2月看作上一年的13月和14月
month += 12;
year--;
}
int century = year / 100;
year %= 100;
int weekDay = year + year / 4 + century / 4 - 2 * century + 26 * (month + 1) / 10 + day - 1;
weekDay %= 7;
if (weekDay < 0) {
weekDay += 7;
}
return weekDay;
}
}
```
运行程序后,输入年份即可输出该年所有月份的日历。
相关推荐
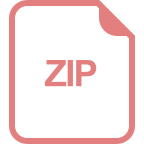
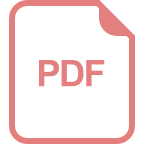














