scrapy爬取宁波的三十天天气预报并用sqlite存储爬取数据
时间: 2023-09-03 14:08:51 浏览: 158
好的,我可以为您提供相关的代码实现,但在开始之前,请确保您已经安装了Scrapy和SQLite。以下是实现步骤:
1. 创建Scrapy项目
在命令行中使用以下命令创建Scrapy项目:
```
scrapy startproject weather
```
2. 创建Spider
在Scrapy项目中创建一个名为 "weather_spider.py" 的Spider。在该Spider中,我们将使用XPath选择器从网站中提取所需数据。以下是示例代码:
```python
import scrapy
from weather.items import WeatherItem
class WeatherSpider(scrapy.Spider):
name = "weather"
allowed_domains = ["tianqi.com"]
start_urls = ["https://www.tianqi.com/ningbo/"]
def parse(self, response):
item = WeatherItem()
item["date"] = response.xpath('//div[@class="day7"]/ul[@class="week"]/li/h1/text()')\
.extract()
item["day_condition"] = response.xpath('//div[@class="day7"]/ul[@class="txt"]/li/p[@class="wea"]/text()')\
.extract()
item["night_condition"] = response.xpath('//div[@class="day7"]/ul[@class="txt"]/li/p[@class="wea"]/text()')\
.extract()
item["high_temp"] = response.xpath('//div[@class="day7"]/ul[@class="txt"]/li/p[@class="tem"]/span/text()')\
.extract()
item["low_temp"] = response.xpath('//div[@class="day7"]/ul[@class="txt"]/li/p[@class="tem"]/i/text()')\
.extract()
yield item
```
在该Spider中,我们从 https://www.tianqi.com/ningbo/ 网站中提取了日期、白天天气状况、晚上天气状况、最高温度和最低温度。
3. 创建Item
在Scrapy项目中创建一个名为 "items.py" 的Item。以下是示例代码:
```python
import scrapy
class WeatherItem(scrapy.Item):
date = scrapy.Field()
day_condition = scrapy.Field()
night_condition = scrapy.Field()
high_temp = scrapy.Field()
low_temp = scrapy.Field()
```
在该Item中,我们定义了从网站中提取的数据字段。
4. 配置Pipeline
在Scrapy项目中创建一个名为 "pipelines.py" 的Pipeline。以下是示例代码:
```python
import sqlite3
class WeatherPipeline(object):
def __init__(self):
self.create_connection()
self.create_table()
def create_connection(self):
self.conn = sqlite3.connect("weather.db")
self.curr = self.conn.cursor()
def create_table(self):
self.curr.execute("""DROP TABLE IF EXISTS weather_tb""")
self.curr.execute("""create table weather_tb(
date text,
day_condition text,
night_condition text,
high_temp text,
low_temp text
)""")
def process_item(self, item, spider):
self.store_db(item)
return item
def store_db(self, item):
self.curr.execute("""insert into weather_tb values (?,?,?,?,?)""", (
item["date"][0],
item["day_condition"][0],
item["night_condition"][0],
item["high_temp"][0],
item["low_temp"][0]
))
self.conn.commit()
```
在该Pipeline中,我们首先创建了SQLite数据库连接和游标。然后,我们定义了一个 "create_table" 函数来创建数据库表。最后,我们定义了一个 "store_db" 函数来将数据存储到数据库中。
5. 配置Settings
在Scrapy项目中的 "settings.py" 文件中添加以下代码:
```python
ITEM_PIPELINES = {'weather.pipelines.WeatherPipeline': 300}
```
该代码将我们创建的Pipeline添加到Scrapy项目中。
6. 运行Spider
在命令行中使用以下命令运行Spider:
```
cd weather
scrapy crawl weather
```
运行完成后,您可以在SQLite数据库中查看存储的数据。
希望这可以帮助您实现所需的功能!
阅读全文
相关推荐
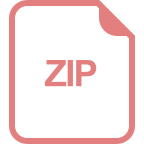
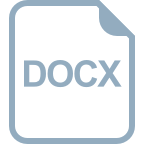
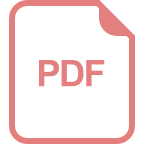















