用宽输入流从一个有中文字符的文本文件中读入所有字符 统计每个字符出现的次数,将统计结果用宽输出流输出到另一个文本文件中
时间: 2024-03-21 07:42:38 浏览: 16
好的,这是一个编程问题。你可以使用 C++ 语言来实现。
首先,你需要使用 `ifstream` 宽输入流从文件中读取所有字符。代码如下:
```c++
#include <fstream>
#include <iostream>
#include <map>
int main() {
std::wifstream ifs("input.txt"); // 打开输入文件
std::wstring content; // 存放文件内容的字符串
if (ifs.is_open()) {
// 读取文件内容
std::wstring line;
while (std::getline(ifs, line)) {
content += line;
}
ifs.close(); // 关闭输入文件
} else {
std::wcerr << L"无法打开输入文件!" << std::endl;
return 1;
}
}
```
接下来,你需要统计每个字符出现的次数。可以使用 `std::map` 来存储字符和出现次数的对应关系。代码如下:
```c++
std::map<wchar_t, int> count; // 存放字符出现次数的map
for (wchar_t c : content) {
count[c]++;
}
```
最后,你需要使用 `ofstream` 宽输出流将统计结果输出到文件中。代码如下:
```c++
std::wofstream ofs("output.txt"); // 打开输出文件
if (ofs.is_open()) {
// 输出统计结果
for (const auto& pair : count) {
ofs << pair.first << L":" << pair.second << std::endl;
}
ofs.close(); // 关闭输出文件
} else {
std::wcerr << L"无法打开输出文件!" << std::endl;
return 1;
}
return 0;
```
完整的代码如下:
```c++
#include <fstream>
#include <iostream>
#include <map>
int main() {
std::wifstream ifs("input.txt"); // 打开输入文件
std::wstring content; // 存放文件内容的字符串
if (ifs.is_open()) {
// 读取文件内容
std::wstring line;
while (std::getline(ifs, line)) {
content += line;
}
ifs.close(); // 关闭输入文件
} else {
std::wcerr << L"无法打开输入文件!" << std::endl;
return 1;
}
std::map<wchar_t, int> count; // 存放字符出现次数的map
for (wchar_t c : content) {
count[c]++;
}
std::wofstream ofs("output.txt"); // 打开输出文件
if (ofs.is_open()) {
// 输出统计结果
for (const auto& pair : count) {
ofs << pair.first << L":" << pair.second << std::endl;
}
ofs.close(); // 关闭输出文件
} else {
std::wcerr << L"无法打开输出文件!" << std::endl;
return 1;
}
return 0;
}
```
希望这个代码能够帮到你!
相关推荐
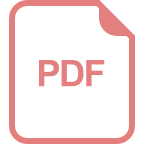
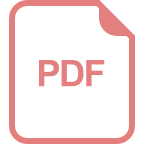
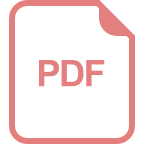














