C语言 编程:数组存储3名同学5门课程成绩 输出最好成绩及所在的行和列 要求:将输入、查找和打印的功能编写成函数 并将二维数组通过指针参数传递的方式由主函数传递到子函数中 输入格式: 每行输入一个同学的5门课的成绩,每个成绩之间空一格,见输入样例 输出格式: 输出共3行: 第一行输出,如:最高分是:97 第二行输出最高分所在的行数,如:行数是:2 第二行输出最高分所在的列数,如:列数是:3
时间: 2023-11-27 09:55:28 浏览: 470
下面是完整的C语言代码:
```c
#include <stdio.h>
void input_scores(int (*scores)[5], int n);
int find_max_score(int (*scores)[5], int n, int *row, int *col);
void print_result(int max_score, int row, int col);
int main()
{
int scores[3][5];
input_scores(scores, 3);
int row, col;
int max_score = find_max_score(scores, 3, &row, &col);
print_result(max_score, row, col);
return 0;
}
// 输入成绩
void input_scores(int (*scores)[5], int n)
{
printf("请输入3位同学的5门课成绩:\n");
for (int i = 0; i < n; i++)
{
printf("第%d位同学:", i+1);
for (int j = 0; j < 5; j++)
{
scanf("%d", &scores[i][j]);
}
}
}
// 查找最高分
int find_max_score(int (*scores)[5], int n, int *row, int *col)
{
int max_score = scores[0][0];
*row = *col = 0;
for (int i = 0; i < n; i++)
{
for (int j = 0; j < 5; j++)
{
if (scores[i][j] > max_score)
{
max_score = scores[i][j];
*row = i;
*col = j;
}
}
}
return max_score;
}
// 输出结果
void print_result(int max_score, int row, int col)
{
printf("最高分是:%d\n", max_score);
printf("行数是:%d\n", row+1);
printf("列数是:%d\n", col+1);
}
```
代码解释:
- `input_scores` 函数用于输入成绩,通过指针参数传递二维数组;
- `find_max_score` 函数用于查找最高分,返回最高分值并通过指针参数返回最高分所在的行和列;
- `print_result` 函数用于输出结果,接收最高分、行和列作为参数。
在主函数中,我们首先定义了一个二维数组 `scores` 来存储成绩。然后调用 `input_scores` 函数输入成绩,再调用 `find_max_score` 函数查找最高分,并将最高分所在的行和列保存在变量 `row` 和 `col` 中。最后调用 `print_result` 函数输出结果。
阅读全文
相关推荐

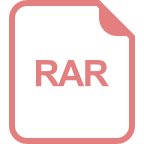
















