实现一个“抽奖小程序”,提供特等奖、一等奖、二等奖及三等奖 4 种等级的抽奖,要 求每种奖项中奖概率不一样,要合理,且不同奖项设置不同的奖品;至少提供抽奖规则 查询、抽奖结果查询和抽奖功能。java
时间: 2024-02-13 19:04:56 浏览: 29
好的,这是一个比较有趣的项目,我们可以使用 Java 编程语言来实现这个“抽奖小程序”。
首先,我们需要设计一个抽奖规则类,这个类可以包含以下属性:
- 奖项名称
- 奖品名称
- 中奖概率
这个类可以使用如下的代码实现:
```java
public class LotteryRule {
private String prizeLevel; // 奖项名称
private String prizeName; // 奖品名称
private double winProbability; // 中奖概率
public LotteryRule(String prizeLevel, String prizeName, double winProbability) {
this.prizeLevel = prizeLevel;
this.prizeName = prizeName;
this.winProbability = winProbability;
}
// getters and setters
}
```
接下来,我们需要设计一个抽奖类,这个类可以包含以下属性:
- 抽奖规则列表
- 中奖结果
这个类可以使用如下的代码实现:
```java
import java.util.ArrayList;
import java.util.List;
import java.util.Random;
public class Lottery {
private List<LotteryRule> rules; // 抽奖规则列表
private String result; // 中奖结果
public Lottery() {
this.rules = new ArrayList<>();
this.result = "";
}
// 添加抽奖规则
public void addRule(LotteryRule rule) {
this.rules.add(rule);
}
// 获取抽奖规则列表
public List<LotteryRule> getRules() {
return this.rules;
}
// 抽奖
public void draw() {
double randomNum = new Random().nextDouble();
double sumProbability = 0.0;
for (LotteryRule rule : this.rules) {
sumProbability += rule.getWinProbability();
if (randomNum < sumProbability) {
this.result = rule.getPrizeName();
break;
}
}
}
// 获取中奖结果
public String getResult() {
return this.result;
}
}
```
这个抽奖类中,我们使用了一个随机数生成器来产生一个随机数,然后根据抽奖规则列表中的中奖概率来确定中奖结果。
最后,我们可以编写一个测试类,来测试这个抽奖程序的功能:
```java
public class LotteryTest {
public static void main(String[] args) {
Lottery lottery = new Lottery();
// 添加抽奖规则
lottery.addRule(new LotteryRule("特等奖", "iPhone 12 Pro Max", 0.01));
lottery.addRule(new LotteryRule("一等奖", "iPad Pro", 0.05));
lottery.addRule(new LotteryRule("二等奖", "AirPods Pro", 0.10));
lottery.addRule(new LotteryRule("三等奖", "优惠券", 0.20));
// 抽奖
lottery.draw();
// 输出中奖结果
System.out.println("恭喜你获得了:" + lottery.getResult());
}
}
```
这个测试类中,我们首先创建了一个 Lottery 对象,并添加了四个抽奖规则,然后调用了 draw() 方法进行抽奖,并输出了中奖结果。
这样,一个简单的“抽奖小程序”就完成了。当然,这只是一个简单的实现,如果要实现更复杂的功能,还需要进一步的完善。
相关推荐
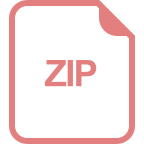
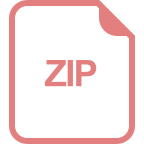














