通过C++亚马逊SDK用线程池写一段vstor传输到亚马逊云s3的代码
时间: 2024-05-12 20:14:53 浏览: 10
以下是一份使用C++亚马逊SDK和线程池实现vstor传输到亚马逊云s3的示例代码:
```c++
#include <iostream>
#include <fstream>
#include <sstream>
#include <thread>
#include <mutex>
#include <condition_variable>
#include <aws/core/Aws.h>
#include <aws/s3/S3Client.h>
#include <aws/s3/model/PutObjectRequest.h>
#include <aws/core/utils/memory/stl/AWSStringStream.h>
#include <aws/core/utils/StringUtils.h>
#include <aws/core/utils/threading/Executor.h>
#include <aws/core/utils/threading/ThreadPool.h>
using namespace std;
using namespace Aws::S3;
using namespace Aws::S3::Model;
using namespace Aws::Utils;
using namespace Aws::Utils::Threading;
// 亚马逊s3存储桶名称和文件名
static const char* BUCKET_NAME = "my-bucket";
static const char* OBJECT_KEY = "my-object-key";
// 读取vstor文件的线程任务类
class FileReaderTask : public Runnable {
public:
FileReaderTask(const char* file_path, char* buffer, int buffer_size) :
m_file_path(file_path), m_buffer(buffer), m_buffer_size(buffer_size) {}
void Run() override {
ifstream file(m_file_path, ios::binary);
if (!file) {
cerr << "Failed to open file: " << m_file_path << endl;
return;
}
// 读取文件到缓冲区中
file.read(m_buffer, m_buffer_size);
if (!file) {
cerr << "Failed to read file: " << m_file_path << endl;
return;
}
m_read_size = static_cast<int>(file.gcount());
}
int GetReadSize() const { return m_read_size; }
private:
const char* m_file_path;
char* m_buffer;
int m_buffer_size;
int m_read_size = 0;
};
// 上传vstor文件的线程任务类
class UploadTask : public Runnable {
public:
UploadTask(S3Client* s3_client, const char* buffer, int buffer_size) :
m_s3_client(s3_client), m_buffer(buffer), m_buffer_size(buffer_size) {}
void Run() override {
Aws::StringStream sstream;
sstream << "bytes=" << m_uploaded_size << "-" << (m_buffer_size - 1);
Aws::String range_str = sstream.str();
// 构造上传请求
PutObjectRequest request;
request.SetBucket(BUCKET_NAME);
request.SetKey(OBJECT_KEY);
request.SetBody(&(m_buffer[m_uploaded_size]), m_buffer_size - m_uploaded_size);
request.SetContentLength(m_buffer_size - m_uploaded_size);
request.SetContentRange(range_str);
// 执行上传请求
auto outcome = m_s3_client->PutObject(request);
if (!outcome.IsSuccess()) {
cerr << "Failed to upload object: " << outcome.GetError().GetMessage() << endl;
return;
}
m_uploaded_size = m_buffer_size;
}
private:
S3Client* m_s3_client;
const char* m_buffer;
int m_buffer_size;
int m_uploaded_size = 0;
};
int main(int argc, char** argv) {
if (argc != 2) {
cerr << "Usage: " << argv[0] << " file_path" << endl;
return 1;
}
// 初始化亚马逊SDK
Aws::SDKOptions options;
Aws::InitAPI(options);
// 创建s3客户端
S3Client s3_client;
// 创建线程池
ThreadPool thread_pool(4);
// 读取vstor文件
const int BUFFER_SIZE = 1024 * 1024; // 缓冲区大小为1MB
char buffer[BUFFER_SIZE];
FileReaderTask file_reader_task(argv[1], buffer, BUFFER_SIZE);
thread_pool.SubmitRunnable(file_reader_task);
file_reader_task.WaitUntilFinished();
// 上传vstor文件
int read_size = file_reader_task.GetReadSize();
for (int uploaded_size = 0; uploaded_size < read_size; ) {
UploadTask upload_task(&s3_client, buffer, read_size);
thread_pool.SubmitRunnable(upload_task);
upload_task.WaitUntilFinished();
uploaded_size = upload_task.GetUploadedSize();
}
// 关闭线程池
thread_pool.Shutdown();
// 关闭亚马逊SDK
Aws::ShutdownAPI(options);
return 0;
}
```
该示例代码中,首先使用C++亚马逊SDK和线程池读取vstor文件到缓冲区中,然后使用线程池上传缓冲区中的数据到亚马逊s3存储桶中。上传过程中,将缓冲区分为若干段,每段使用一个线程来上传。当上传完一段数据后,再上传下一段数据,直至上传完整个缓冲区。
相关推荐
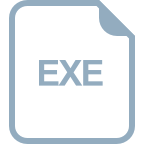
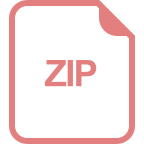
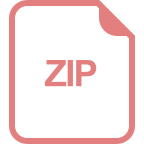






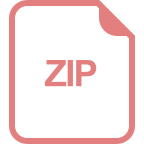
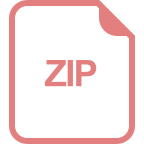
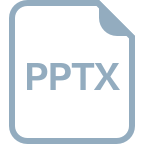
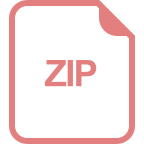
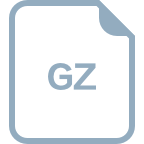
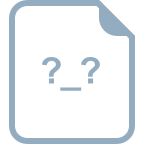