为什么MLP模型生成的数据和数据集数量级差很多
时间: 2023-03-28 15:00:59 浏览: 69
MLP模型生成的数据和数据集数量级差很多可能是因为模型的训练数据不足或者模型的参数设置不合理。在训练模型时,需要尽可能多地提供数据,以便模型能够更好地学习数据的特征。此外,还需要对模型的参数进行调整,以便使其更好地适应数据集。
相关问题
MLP多层感知模型 代码 Python mnist数据集
以下是一个简单的 Python 代码示例,用于使用 MLP 多层感知模型训练和测试 MNIST 数据集。
首先,需要导入所需的库和模块:
```python
import numpy as np
import matplotlib.pyplot as plt
from keras.datasets import mnist
from keras.models import Sequential
from keras.layers import Dense, Dropout
from keras.optimizers import RMSprop
```
然后,加载 MNIST 数据集并将其分为训练集和测试集:
```python
(x_train, y_train), (x_test, y_test) = mnist.load_data()
```
接下来,对数据进行预处理,将图像数据转换为 1D 向量并将其归一化:
```python
x_train = x_train.reshape(60000, 784)
x_test = x_test.reshape(10000, 784)
x_train = x_train.astype('float32')
x_test = x_test.astype('float32')
x_train /= 255
x_test /= 255
```
然后,将标签数据进行 one-hot 编码:
```python
y_train = keras.utils.to_categorical(y_train, num_classes)
y_test = keras.utils.to_categorical(y_test, num_classes)
```
定义 MLP 模型:
```python
model = Sequential()
model.add(Dense(512, activation='relu', input_shape=(784,)))
model.add(Dropout(0.2))
model.add(Dense(512, activation='relu'))
model.add(Dropout(0.2))
model.add(Dense(num_classes, activation='softmax'))
```
编译模型并进行训练:
```python
model.compile(loss='categorical_crossentropy',
optimizer=RMSprop(),
metrics=['accuracy'])
history = model.fit(x_train, y_train,
batch_size=batch_size,
epochs=epochs,
verbose=1,
validation_data=(x_test, y_test))
```
最后,评估模型并绘制准确率和损失曲线:
```python
score = model.evaluate(x_test, y_test, verbose=0)
print('Test loss:', score[0])
print('Test accuracy:', score[1])
# 绘制准确率和损失曲线
plt.plot(history.history['accuracy'], label='accuracy')
plt.plot(history.history['val_accuracy'], label='val_accuracy')
plt.plot(history.history['loss'], label='loss')
plt.plot(history.history['val_loss'], label='val_loss')
plt.title('Training and validation accuracy and loss')
plt.xlabel('Epoch')
plt.ylabel('Accuracy/Loss')
plt.legend()
plt.show()
```
完整的代码示例:
```python
import numpy as np
import matplotlib.pyplot as plt
from keras.datasets import mnist
from keras.models import Sequential
from keras.layers import Dense, Dropout
from keras.optimizers import RMSprop
# 设置参数
batch_size = 128
num_classes = 10
epochs = 20
# 加载数据集
(x_train, y_train), (x_test, y_test) = mnist.load_data()
# 数据预处理
x_train = x_train.reshape(60000, 784)
x_test = x_test.reshape(10000, 784)
x_train = x_train.astype('float32')
x_test = x_test.astype('float32')
x_train /= 255
x_test /= 255
# 标签 one-hot 编码
y_train = keras.utils.to_categorical(y_train, num_classes)
y_test = keras.utils.to_categorical(y_test, num_classes)
# 定义模型
model = Sequential()
model.add(Dense(512, activation='relu', input_shape=(784,)))
model.add(Dropout(0.2))
model.add(Dense(512, activation='relu'))
model.add(Dropout(0.2))
model.add(Dense(num_classes, activation='softmax'))
# 编译模型并训练
model.compile(loss='categorical_crossentropy',
optimizer=RMSprop(),
metrics=['accuracy'])
history = model.fit(x_train, y_train,
batch_size=batch_size,
epochs=epochs,
verbose=1,
validation_data=(x_test, y_test))
# 评估模型
score = model.evaluate(x_test, y_test, verbose=0)
print('Test loss:', score[0])
print('Test accuracy:', score[1])
# 绘制准确率和损失曲线
plt.plot(history.history['accuracy'], label='accuracy')
plt.plot(history.history['val_accuracy'], label='val_accuracy')
plt.plot(history.history['loss'], label='loss')
plt.plot(history.history['val_loss'], label='val_loss')
plt.title('Training and validation accuracy and loss')
plt.xlabel('Epoch')
plt.ylabel('Accuracy/Loss')
plt.legend()
plt.show()
```
MLP-MNIST数据集
MLP-MNIST数据集是一个用于手写数字识别的数据集,其中包含了一系列的手写数字图像和相应的标签。每个图像都是一个28x28像素的灰度图像,标签表示了图像所代表的数字。
为了对MLP-MNIST数据集进行处理和分析,以下是一些常见的步骤和方法:
1. 导入数据集:首先,我们需要从数据集中读取图像和标签数据。这可以通过使用适当的库和函数来完成,例如使用Python的NumPy库或者深度学习框架如TensorFlow或PyTorch提供的接口。
2. 数据预处理:在使用MLP模型之前,通常需要对数据进行一些预处理操作。这可能包括将图像数据转换为适当的张量形式,对图像进行标准化处理以确保数据的一致性和可比性,还可以将标签进行独热编码以适应模型的输出要求。
3. 定义模型结构:MLP-MNIST数据集通常使用多层感知机(MLP)模型进行训练和预测。这种模型是一种前馈神经网络,由多个全连接层组成。可以定义不同层的神经元数量、激活函数和其他参数来适应具体的任务。
4. 初始化模型参数:在训练模型之前,需要初始化模型的参数。这些参数可以是权重和偏置,通过随机初始化或者使用特定的初始化方法来获取合适的初始值。
5. 定义激活函数:对于MLP模型的隐含层,通常需要使用激活函数对输出进行非线性变换。常见的激活函数包括ReLU、Sigmoid和Tanh等,它们可以增加模型的表达能力和非线性拟合能力。
6. 防止过拟合:在使用带有隐含层的MLP模型时,过拟合是一个常见的问题。为了解决这个问题,可以采用一些技术来防止过拟合,如正则化方法(如权重衰减)和丢弃法(Dropout)等。
综上所述,处理和分析MLP-MNIST数据集的一般步骤包括导入数据集、数据预处理、定义模型结构、初始化模型参数、定义激活函数以及防止过拟合。这些步骤可以帮助我们构建一个用于手写数字识别的MLP模型,并进行训练和预测。
相关推荐
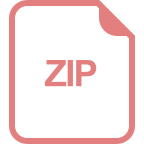
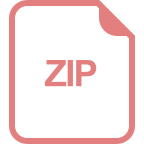
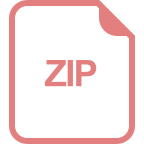












