代码优化: dst = item[1] if dst == 'null' or dst == '' or dst == ' ': dst = -999999
时间: 2024-02-07 13:01:52 浏览: 217
可以将多个判断语句合并为一个条件判断,如下所示:
```
dst = item[1]
if not dst or dst.strip() == 'null':
dst = -999999
```
这里使用了 Python 中的`not`关键字来判断`dst`是否为空或为`None`,同时使用`strip()`方法去除字符串两端的空格,避免只有空格的情况被误判。这样可以使代码更加简洁和易读。
相关问题
def point(dst,roll): color = dst[roll] black_count = np.sum(color == 0) if black_count == 0: return 0 else: black_index = np.where(color == 0) return black_index[0][0] while (1): ret, frame = cap.read() cv2.imshow("recognize_face", frame) # 转化为灰度图 gray = cv2.cvtColor(frame, cv2.COLOR_BGR2GRAY) # 大津法二值化 retval, dst = cv2.threshold(gray, 0, 255, cv2.THRESH_OTSU) # 膨胀,白区域变大 dst = cv2.dilate(dst, None, iterations=2) # # 腐蚀,白区域变小 # dst = cv2.erode(dst, None, iterations=6) dst = dst.transpose() image = dst image = cv2.flip(dst,0,dst=None) cv2.imshow("灰度图", image) # 单看某行的像素值 y1 = point(dst,500) y2 = point(dst,300) y3 = point(dst,600) direction_line = y3-y2 if y2==0 or y3==0 : direction_line = 0 direction = y1-200 if cv2.waitKey(1) & 0xFF == ord('q'): break distance = Distance_test() if distance < 50: stop() print("stoping") else: run(direction*0.1,direction_line)
这是一个在树莓派(Raspberry Pi)上使用OpenCV库进行图像处理,结合超声波测距和电机控制的小车控制程序。这个程序的主要逻辑是:循环读取摄像头数据并在窗口中显示,将图像转化为灰度图并进行二值化、膨胀、腐蚀等处理,然后根据某些行的像素值计算出行进方向和方向偏移量,并根据超声波传感器的距离值控制小车的运动。
具体来说,程序中定义了一个名为point()的函数,用于计算某一行像素值中黑色像素的位置。然后在主程序中循环调用该函数计算不同行的像素值,并根据这些值计算出行进方向和方向偏移量。此外,程序还调用Distance_test()函数获取超声波传感器的距离值,并根据距离值控制小车的运动,如果距离小于50,则停车,否则根据行进方向和方向偏移量控制小车前进。
需要注意的是,程序中使用了一些OpenCV库中的图像处理函数,如cvtColor()、threshold()、dilate()等,用于对图像进行处理。同时,程序还需要连接超声波传感器和电机驱动模块等硬件设备,才能实现完整的小车控制功能。
解释此代码 def sobel_gradient(src): src = gauss_filter(src, 5, 3) height, width = len(src), len(src[0]) dst = [[0] * width for _ in range(height)] gx = [[-1,-2,-1],[0,0,0],[1,2,1]] gy =[[-1,0,1],[-2,0,2],[-1,0,1]] for i in range(1, height-1): for j in range(1, width-1): sum_gx = 0 sum_gy = 0 for m in range(-1, 2): for n in range(-1, 2): if i+m >= 0 and i+m < height and j+n >= 0 and j+n < width: sum_gx += gx[m+1][n+1] * src[i+m][j+n] sum_gy += gy[m+1][n+1] * src[i+m][j+n] dst[i][j] = abs(sum_gx) + abs(sum_gy) if dst[i][j] >= 70: dst[i][j] = dst[i][j]+200 else: dst[i][j] = 0 #dst = np.round(dst).astype(np.uint8) for i in range(height): for j in range(width): dst[i][j] = int(max(min(dst[i][j], 255), 0)) return dst
这段代码实现了Sobel算子的梯度计算函数。我将逐行解释代码的功能:
1. 首先调用 `gauss_filter()` 函数对输入图像 `src` 进行高斯滤波处理,滤波器大小为5,标准差为3。高斯滤波的目的是对图像进行平滑处理,以减少噪声的影响。
2. 获取输入图像的高度和宽度:`height, width = len(src), len(src[0])`。
3. 创建一个与输入图像大小相同的输出图像数组 `dst`,并初始化为全零。
4. 定义Sobel算子的水平和垂直滤波器模板:`gx` 和 `gy`。这两个滤波器模板用于计算图像中每个像素点的水平和垂直梯度。
5. 遍历输入图像中除边缘区域外的每个像素点:对于每个像素点,计算其周围像素与Sobel模板的卷积,并根据卷积结果计算梯度大小。
6. 在计算梯度大小时,首先定义两个变量 `sum_gx` 和 `sum_gy` 分别用于累加水平和垂直方向的梯度。然后使用两个嵌套循环遍历Sobel模板中的每个元素,将模板与输入图像的对应位置进行点乘并累加到 `sum_gx` 和 `sum_gy` 中。
7. 计算梯度大小的公式为 `dst[i][j] = abs(sum_gx) + abs(sum_gy)`。这里使用绝对值将梯度值转换为正数。
8. 根据梯度大小进行阈值处理:如果梯度值超过阈值70,则将其增加200;否则将其设为0。这个阈值处理可以用来增强边缘特征。
9. 使用两个嵌套循环遍历输出图像 `dst` 中的每个像素,将像素值限制在0到255之间。
10. 返回输出图像 `dst`。
总之,这段代码实现了Sobel算子的梯度计算,用于检测图像中的边缘特征。它首先对输入图像进行高斯滤波,然后使用Sobel模板计算每个像素点的梯度大小,并根据阈值对梯度进行处理,最后返回处理后的输出图像。
阅读全文
相关推荐
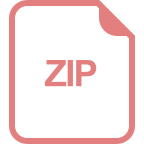
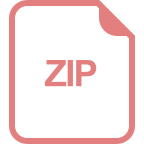
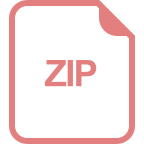










