maven javax.crypto包依赖
时间: 2023-10-10 10:06:48 浏览: 133
你可以在 Maven 配置文件 pom.xml 中添加以下依赖来使用 javax.crypto 包:
```xml
<dependency>
<groupId>javax.crypto</groupId>
<artifactId>javax.crypto-api</artifactId>
<version>1.1.1</version>
</dependency>
```
这将向你的项目添加 javax.crypto 包及其依赖项。请注意,这个依赖项只提供了 javax.crypto API 的接口,如果你需要实现它们的具体算法,你需要添加另一个依赖项。
例如,如果你需要使用 AES 算法,你可以添加以下依赖项:
```xml
<dependency>
<groupId>org.bouncycastle</groupId>
<artifactId>bcprov-jdk15on</artifactId>
<version>1.68</version>
</dependency>
```
这将向你的项目添加 Bouncy Castle 提供的 AES 实现。
相关问题
国密算法(SM2-SM3)Java 使用 demo maven 工程
很高兴回答你的问题!以下是一个使用国密算法(SM2-SM3)的Java Demo Maven工程,供您参考:
1. 首先,在pom.xml文件中添加以下依赖:
```xml
<dependency>
<groupId>com.huawei.security</groupId>
<artifactId>sm</artifactId>
<version>2.0.5.300</version>
</dependency>
```
2. 创建SM2密钥对
```java
import com.huawei.security.sm.SM2KeyPair;
import com.huawei.security.sm.SM2PrivateKey;
import com.huawei.security.sm.SM2PublicKey;
import java.security.NoSuchAlgorithmException;
import java.security.NoSuchProviderException;
import java.security.Security;
public class SM2Demo {
public static void main(String[] args) throws NoSuchProviderException, NoSuchAlgorithmException {
Security.addProvider(new org.bouncycastle.jce.provider.BouncyCastleProvider());
SM2KeyPair sm2KeyPair = new SM2KeyPair();
SM2PublicKey publicKey = sm2KeyPair.getPublicKey();
SM2PrivateKey privateKey = sm2KeyPair.getPrivateKey();
System.out.println("publicKey: " + publicKey.getHexString());
System.out.println("privateKey: " + privateKey.getHexString());
}
}
```
3. SM2加密和解密
```java
import com.huawei.security.sm.SM2PrivateKey;
import com.huawei.security.sm.SM2PublicKey;
import com.huawei.security.sm.SM2Engine;
import java.security.NoSuchAlgorithmException;
import java.security.NoSuchProviderException;
import java.security.Security;
import java.security.InvalidAlgorithmParameterException;
import java.security.InvalidKeyException;
import java.security.KeyPairGenerator;
import javax.crypto.Cipher;
import javax.crypto.NoSuchPaddingException;
public class SM2Demo {
public static void main(String[] args) throws NoSuchProviderException, NoSuchAlgorithmException, InvalidAlgorithmParameterException, InvalidKeyException, NoSuchPaddingException {
Security.addProvider(new org.bouncycastle.jce.provider.BouncyCastleProvider());
// 创建密钥对
KeyPairGenerator keyPairGenerator = KeyPairGenerator.getInstance("EC", "BC");
keyPairGenerator.initialize(256);
SM2KeyPair sm2KeyPair = new SM2KeyPair(keyPairGenerator.generateKeyPair());
// 获取公钥和私钥
SM2PublicKey publicKey = sm2KeyPair.getPublicKey();
SM2PrivateKey privateKey = sm2KeyPair.getPrivateKey();
// 加密
SM2Engine sm2Engine = new SM2Engine();
Cipher cipher = Cipher.getInstance(sm2Engine.getAlgorithmName(), "BC");
cipher.init(Cipher.ENCRYPT_MODE, publicKey);
byte[] plaintext = "Hello, world!".getBytes();
byte[] ciphertext = cipher.doFinal(plaintext);
System.out.println("ciphertext: " + new String(ciphertext));
// 解密
cipher.init(Cipher.DECRYPT_MODE, privateKey);
byte[] decrypted = cipher.doFinal(ciphertext);
System.out.println("decrypted: " + new String(decrypted));
}
}
```
4. SM3哈希算法
```java
import com.huawei.security.sm.SM3Digest;
import java.security.NoSuchAlgorithmException;
import java.security.NoSuchProviderException;
import java.security.Security;
public class SM3Demo {
public static void main(String[] args) throws NoSuchProviderException, NoSuchAlgorithmException {
Security.addProvider(new org.bouncycastle.jce.provider.BouncyCastleProvider());
// 计算哈希值
SM3Digest sm3Digest = new SM3Digest();
byte[] plaintext = "Hello, world!".getBytes();
sm3Digest.update(plaintext, 0, plaintext.length);
byte[] hash = new byte[sm3Digest.getDigestSize()];
sm3Digest.doFinal(hash, 0);
System.out.println("hash: " + org.bouncycastle.util.encoders.Hex.toHexString(hash));
}
}
```
希望这个Demo工程能够帮助您使用国密算法(SM2-SM3)的Java开发。如果您有任何疑问,请随时联系我!
具体一点,哪个包哪个java文件
在Java中,通常使用第三方库来生成和验证Token,常用的有JWT和OAuth2。下面是一个使用JWT生成和验证Token的示例代码:
1. 首先,需要在Maven或Gradle中添加JWT依赖:
Maven:
```xml
<dependency>
<groupId>io.jsonwebtoken</groupId>
<artifactId>jjwt</artifactId>
<version>0.9.1</version>
</dependency>
```
Gradle:
```groovy
implementation 'io.jsonwebtoken:jjwt:0.9.1'
```
2. 接着,创建一个TokenUtil类,用于生成和验证Token:
TokenUtil.java:
```java
import io.jsonwebtoken.Claims;
import io.jsonwebtoken.Jwts;
import io.jsonwebtoken.SignatureAlgorithm;
import io.jsonwebtoken.security.Keys;
import javax.crypto.SecretKey;
import java.util.Date;
import java.util.HashMap;
import java.util.Map;
public class TokenUtil {
private static final String SECRET_KEY = "secret-key"; // 设置密钥
// 生成Token
public static String generateToken(String subject, long ttlMillis) {
SecretKey key = Keys.hmacShaKeyFor(SECRET_KEY.getBytes());
Map<String, Object> claims = new HashMap<>();
return Jwts.builder()
.setClaims(claims)
.setSubject(subject)
.setIssuedAt(new Date())
.setExpiration(new Date(System.currentTimeMillis() + ttlMillis))
.signWith(key, SignatureAlgorithm.HS256)
.compact();
}
// 验证Token
public static String validateToken(String token) {
try {
Claims claims = Jwts.parserBuilder()
.setSigningKey(SECRET_KEY.getBytes())
.build()
.parseClaimsJws(token)
.getBody();
return claims.getSubject();
} catch (Exception e) {
return null;
}
}
}
```
在上面的代码中,`generateToken`函数接受一个主题(subject)和有效期(ttlMillis)作为参数,生成一个包含主题和时间戳的payload,并使用密钥生成一个Token,有效期为指定的时间。`validateToken`函数接受一个Token作为参数,使用密钥验证Token的有效性,并返回主题。在使用时,可以调用`generateToken`生成Token,然后将其返回给客户端,后续请求时将Token添加到请求头中,在服务器端使用`validateToken`验证Token的有效性并获取主题。
这个代码应该放在后端服务端的Java文件中,例如在一个名为TokenUtil.java的类中。
相关推荐







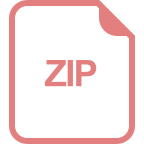
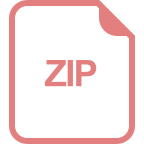
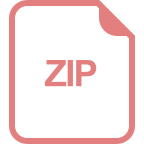
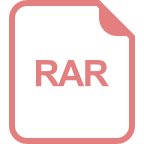