封装一个 HTTP 请求的模块,使用了 Vue.js 框架和 uni-app 应用开发框架
时间: 2024-05-18 09:18:38 浏览: 106
好的,下面是封装 HTTP 请求的模块:
```javascript
// http.js
import Vue from 'vue'
import uni from 'uni-app'
// 全局默认配置
const DEFAULT_OPTIONS = {
baseURL: 'https://example.com', // 接口基础路径
timeout: 5000, // 请求超时时间
headers: {
'Content-Type': 'application/json', // 默认请求头
},
responseType: 'json', // 响应类型
withCredentials: false, // 是否允许跨域携带 cookie
}
// 创建 axios 实例
const axios = uni.request.create(DEFAULT_OPTIONS)
// 请求拦截器
axios.interceptors.request.use(
config => {
// 在发送请求之前做些什么
const token = Vue.$cookies.get('token') // 从 cookie 中读取 token
if (token) {
config.headers.Authorization = `Bearer ${token}` // 添加授权头
}
return config
},
error => {
// 对请求错误做些什么
return Promise.reject(error)
}
)
// 响应拦截器
axios.interceptors.response.use(
response => {
// 对响应数据做些什么
return response.data
},
error => {
// 对响应错误做些什么
return Promise.reject(error)
}
)
export default axios
```
以上代码将 `uni.request` 封装成了一个 axios 实例,并配置了请求拦截器和响应拦截器。在请求拦截器中,我们读取了保存在 cookie 中的 token,并添加到请求头中,实现了全局授权。在响应拦截器中,我们只返回了响应数据的 `data` 字段,这样就可以在业务代码中直接使用数据,而不需要写很多重复的代码。
接下来,我们可以在业务代码中使用封装好的 axios 模块,例如:
```javascript
// 使用 http.js 发送 GET 请求
import axios from '@/utils/http'
axios.get('/api/users')
.then(response => {
console.log(response)
})
.catch(error => {
console.error(error)
})
// 使用 http.js 发送 POST 请求
axios.post('/api/login', { username: 'admin', password: '123456' })
.then(response => {
console.log(response)
})
.catch(error => {
console.error(error)
})
```
以上代码演示了如何使用封装好的 axios 模块发送 GET 和 POST 请求,业务代码中只需要关注请求路径和请求参数即可。
阅读全文
相关推荐
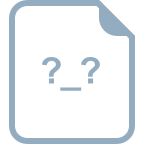
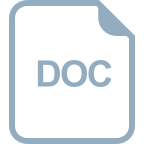
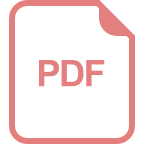
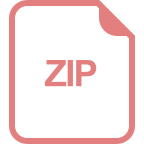
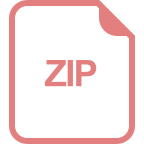
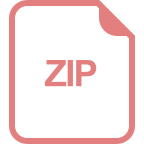
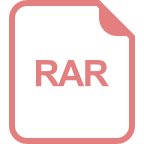
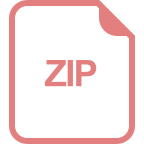
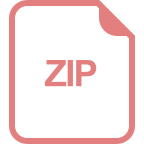
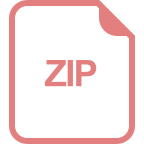
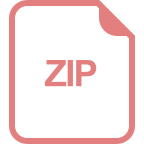
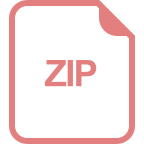
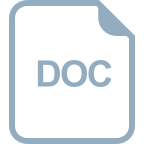



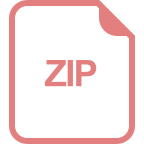