编写 C++程序,定义重载函数 double area(形参表),分别用来求长方形、三角形、正方形、圆的面积,主函数中给定必要的初始数据,分别调用这些函数求面积。
时间: 2024-03-20 20:40:29 浏览: 73
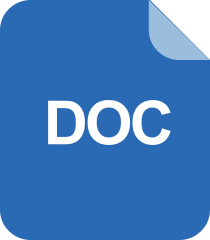
C++编写计算三角形、正方形和圆形面积程序

好的,以下是 C++ 程序的实现:
```c++
#include <iostream>
using namespace std;
// 长方形面积计算函数
double area(double length, double width) {
return length * width;
}
// 三角形面积计算函数
double area(double bottom, double height, char type) {
if (type == 'r' || type == 'R') {
return 0.5 * bottom * height;
}
else if (type == 'i' || type == 'I') {
double inradius = height / 2.0;
double exradius = sqrt(inradius * inradius + (bottom / 2.0) * (bottom / 2.0));
return 0.5 * bottom * exradius;
}
else {
return 0;
}
}
// 正方形面积计算函数
double area(double side) {
return side * side;
}
// 圆形面积计算函数
double area(double radius, char type) {
if (type == 's' || type == 'S') {
return M_PI * radius * radius;
}
else if (type == 'c' || type == 'C') {
return 2 * M_PI * radius;
}
else {
return 0;
}
}
int main() {
double length, width, bottom, height, side, radius;
char type;
// 长方形面积计算
length = 3.5;
width = 2.5;
cout << "长方形面积:" << area(length, width) << endl;
// 三角形面积计算
bottom = 4.0;
height = 3.0;
type = 'r';
cout << "三角形面积:" << area(bottom, height, type) << endl;
// 正方形面积计算
side = 5.0;
cout << "正方形面积:" << area(side) << endl;
// 圆形面积计算
radius = 2.0;
type = 's';
cout << "圆形面积:" << area(radius, type) << endl;
return 0;
}
```
程序中定义了4个面积计算函数,分别用来计算长方形、三角形、正方形、圆的面积。这些函数的形参表略有不同,通过函数重载实现了对不同形状的面积计算。在主函数中,给定了必要的初始数据,并分别调用这些函数求解面积。
需要注意的是,在三角形面积计算函数 `area(double bottom, double height, char type)` 中,通过 `type` 参数来区分不同类型的三角形,其中 'r' 或 'R' 表示直角三角形,'i' 或 'I' 表示等边内切三角形。在计算等边内切三角形面积时,需要先计算出三角形外接圆半径,然后根据海伦公式计算三角形面积。
阅读全文
相关推荐





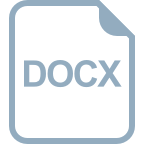
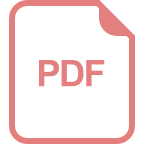
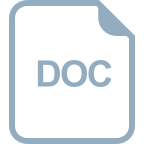
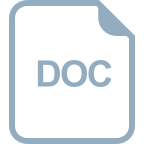
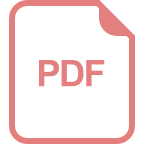
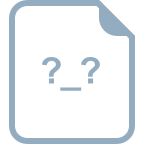
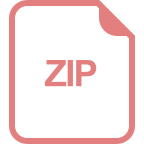
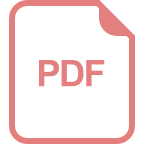
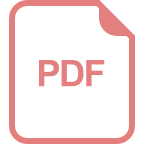