C++中获取微秒的函数是什么
时间: 2024-05-08 08:17:03 浏览: 19
在 C 中获取微秒的函数是 gettimeofday()。它可以在 <sys/time.h> 头文件中找到。该函数返回当前时间的秒数和微秒数,可以用来计算代码执行的时间。以下是一个示例用法:
```
#include <stdio.h>
#include <sys/time.h>
int main() {
struct timeval start, end;
gettimeofday(&start, NULL);
// 执行需要计时的代码
gettimeofday(&end, NULL);
long seconds = end.tv_sec - start.tv_sec;
long microseconds = end.tv_usec - start.tv_usec;
double elapsed = seconds + microseconds*1e-6;
printf("Elapsed time: %.6f seconds\n", elapsed);
return 0;
}
```
相关问题
c++获取时间,单位微秒
你可以使用C++标准库中的chrono库来获取时间,包括微秒。下面是一个示例代码:
```c++
#include <chrono>
#include <iostream>
int main() {
auto start = std::chrono::steady_clock::now();
// 执行需要计时的代码
auto end = std::chrono::steady_clock::now();
auto diff = end - start;
std::cout << "Time elapsed: " << std::chrono::duration_cast<std::chrono::microseconds>(diff).count() << " microseconds" << std::endl;
return 0;
}
```
在这个示例中,我们使用了steady_clock来获取当前时间,并且使用了duration_cast函数将时间差转换为微秒。
c++中可以直接使用的延迟函数
在C++中,可以使用 `<chrono>` 头文件中的 `std::this_thread::sleep_for` 函数来实现延迟。该函数需要一个时间参数,表示要延迟的时间长度。下面是一个使用示例:
```cpp
#include <iostream>
#include <chrono>
#include <thread>
int main() {
std::cout << "Start" << std::endl;
// 延迟1秒
std::this_thread::sleep_for(std::chrono::seconds(1));
std::cout << "End" << std::endl;
return 0;
}
```
在上面的示例中,`std::this_thread::sleep_for(std::chrono::seconds(1))` 会使程序暂停1秒钟。你可以根据需要传递不同的时间参数,如 `std::chrono::milliseconds` 表示毫秒,`std::chrono::microseconds` 表示微秒等。
相关推荐
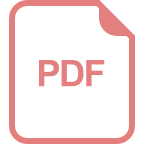
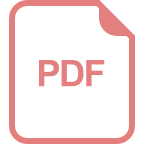
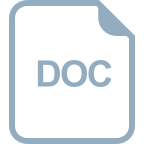












