#include <stdio.h> #include <string.h> #define MAX 100 void fun(char (*a)[81],int num,char **max) { int i=0; *max=a[0]; for(i=0;i<num;i++) if(strlen(*max)<strlen(a[i])) *max=a[i]; } int main() { char ss[10][81],*ps; int n,i=0; printf("输入若干各字符串:"); gets(ss[i]); puts(ss[i]); while(!strcmp(ss[i],"****")==0) { i++; gets(ss[i]); puts(ss[i]); } n=i; fun(ss,n,&ps); printf("\nmax=%s\n",ps); printf("\n"); }
时间: 2024-04-20 09:27:06 浏览: 24
这段代码是一个求字符串数组中最长字符串的程序,使用的是指针和函数传参的方式。
函数 fun 的参数为 char (*a)[81],表示一个长度为 81 的字符数组的指针,即指向一个字符串数组的指针;int num,表示字符串数组中字符串的个数;char **max,表示一个指向字符指针的指针,即指向最长字符串的指针。
在函数中,首先将最长字符串指针指向第一个字符串,然后依次遍历字符串数组,如果发现有比当前最长字符串更长的字符串,就将最长字符串指针指向该字符串。最后返回最长字符串指针。
在主函数中,先定义一个字符数组 ss,用于存储输入的字符串。然后使用 gets 函数逐个读取字符串,遇到字符串 "****" 时结束输入。接着调用函数 fun,传入字符串数组和最长字符串指针的地址,得到最长字符串的指针。最后输出最长字符串即可。
需要注意的是,该程序存在一些问题,gets 函数不安全,容易导致缓冲区溢出,应该使用 fgets 函数代替。另外,如果输入的字符串个数超过了 10 个,程序就会出现数组越界的错误。
相关问题
只使用#include <stdio.h> #include <string.h>
这两个头文件都是C语言标准库中的头文件,其中<stdio.h>包含了一些输入输出函数的声明,如printf和scanf;<string.h>包含了一些字符串操作函数的声明,如strcpy和strlen。使用这两个头文件可以完成很多基本的C语言程序设计,例如输出、输入、字符串操作等等。下面给出一个简单的例子:
```
#include <stdio.h>
#include <string.h>
int main() {
char str1[10] = "hello";
char str2[10] = "world";
printf("%s ", str1);
printf("%s\n", str2);
strcat(str1, str2);
printf("%s\n", str1);
printf("The length of str1 is %d.\n", strlen(str1));
return 0;
}
```
这个程序输出了两个字符串"hello"和"world",然后将它们拼接在一起并输出结果"helloworld",最后输出了拼接后的字符串长度11。
include <stdio.h>#include <stdlib.h>
这是一个C语言的标准头文件的引用,其中<stdio.h>包含了C语言的输入输出函数,而<stdlib.h>包含了一些通用的工具函数和内存分配函数。这些头文件在C语言中非常常见,几乎每个C程序都会用到它们。下面是一个简单的例子,展示了如何使用这两个头文件。
```c
#include <stdio.h>
#include <stdlib.h>
int main()
{
int num;
char str[100];
printf("Enter an integer: ");
scanf("%d", &num);
printf("You entered %d.\n", num);
printf("Enter a string: ");
scanf("%s", str);
printf("You entered %s.\n", str);
return 0;
}
```
上面的程序使用了<stdio.h>中的printf和scanf函数,以及<stdlib.h>中的exit函数。输入一个整数和一个字符串,然后将它们输出到控制台上。
相关推荐
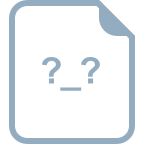
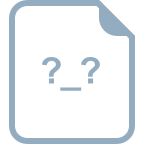













