写一段爬取气象数据的代码
时间: 2023-03-06 10:21:16 浏览: 127
好的,以下是一个简单的爬取气象数据的Python代码:
```python
import requests
from bs4 import BeautifulSoup
# 气象数据所在的网页链接
url = 'https://www.weather.com/'
# 发送请求,获取网页内容
response = requests.get(url)
# 解析网页内容
soup = BeautifulSoup(response.content, 'html.parser')
# 查找气象数据所在的元素,比如温度和天气状况
temperature = soup.find('div', {'class': 'temperature'}).text
weather_condition = soup.find('div', {'class': 'weather-condition'}).text
# 输出气象数据
print(f"当前温度:{temperature}")
print(f"天气状况:{weather_condition}")
```
需要注意的是,这只是一个简单的示例代码,具体爬取气象数据的方法可能因网站而异,需要根据具体情况进行修改。同时,在爬取数据时,需要遵守网站的爬虫协议,不要过于频繁地发送请求,以免给网站带来负担。
相关问题
python爬取台风数据并进行路径可视化处理给出代码
好的!以下是一个简单的示例代码,展示了如何使用 Python 爬取台风数据并进行路径可视化处理。这里我们使用的是中国气象局的台风数据:
```python
import requests
from bs4 import BeautifulSoup
import pandas as pd
import matplotlib.pyplot as plt
import cartopy.crs as ccrs
# 爬取数据
url = 'http://www.nmc.cn/f/rest/passed/typhoon?userId=×tamp=1627634548372'
response = requests.get(url)
soup = BeautifulSoup(response.content, 'html.parser')
# 解析数据
data = []
for item in soup.find_all('item'):
typhoon_id = item.typhoonid.string
typhoon_name = item.typhoonname.string
typhoon_time = item.time.string
typhoon_lat = item.lat.string
typhoon_lon = item.lon.string
typhoon_wind = item.wind.string
typhoon_pressure = item.pressure.string
data.append([typhoon_id, typhoon_name, typhoon_time, typhoon_lat, typhoon_lon, typhoon_wind, typhoon_pressure])
# 转换为 DataFrame 格式
df = pd.DataFrame(data, columns=['id', 'name', 'time', 'lat', 'lon', 'wind', 'pressure'])
df['time'] = pd.to_datetime(df['time']) # 转换日期格式
df['lat'] = pd.to_numeric(df['lat']) / 10 # 转换纬度格式
df['lon'] = pd.to_numeric(df['lon']) / 10 # 转换经度格式
# 绘制地图
fig = plt.figure(figsize=(10, 10))
ax = plt.axes(projection=ccrs.PlateCarree())
ax.stock_img()
ax.coastlines()
# 绘制路径
for name, group in df.groupby('name'):
ax.plot(group['lon'], group['lat'], label=name)
# 添加图例
ax.legend(loc='upper left', fontsize='large')
# 显示图形
plt.show()
```
这段代码会爬取中国气象局的台风数据,并将台风的路径绘制在地图上。需要注意的是,这里使用了 cartopy 库进行地图投影和绘制,需要提前安装。另外,由于中国气象局的数据可能会有更新或者变动,这段代码的运行结果可能与实际情况略有出入。
python爬取呼伦贝尔历史天气
Python爬取网页数据通常会利用诸如`requests`库获取HTML内容,然后使用如`BeautifulSoup`或`lxml`等解析库分析结构并提取所需信息。对于呼伦贝尔历史天气这种特定需求,你需要访问一个提供历史天气数据的网站,比如中国气象局、AccuWeather这类站点。
首先,你需要安装必要的库:
```bash
pip install requests beautifulsoup4 lxml
```
接着,可以编写一段简单的Python脚本示例如下:
```python
import requests
from bs4 import BeautifulSoup
# 指定目标网站URL,替换为你找到的历史天气页面
url = "https://example.com/huhunbeiliehe/weather" # 替换为实际地址
response = requests.get(url)
if response.status_code == 200:
soup = BeautifulSoup(response.text, 'lxml')
# 找到包含历史天气数据的部分,这取决于网站的具体结构
weather_data = soup.find('div', class_='historical_weather') or soup.find('table', id='history_table')
# 提取具体的数据(如日期、温度等),这里需要进一步分析HTML结构
dates = [date.text for date in weather_data.select('.date')]
temperatures = [temp.text for temp in weather_data.select('.temperature')]
# 输出或保存数据
print(f"日期:{dates}, 温度:{temperatures}")
else:
print("无法获取数据,HTTP状态码:", response.status_code)
```
请注意,上述代码仅为示例,实际操作中需要根据目标网站的HTML结构进行调整。在抓取数据前,请确保遵守网站的robots.txt规则,并尊重版权政策。
阅读全文
相关推荐
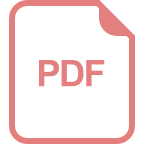
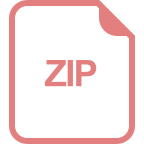
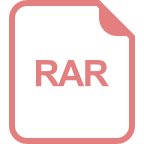
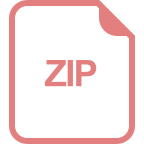
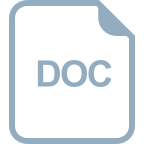
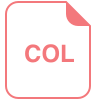
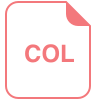


