给我一个详细的基于opencv的车道线检测
时间: 2024-06-08 22:06:08 浏览: 14
好的,以下是一个基于OpenCV的车道线检测的详细步骤:
1. 导入必要的库和读取图像
```python
import cv2
import numpy as np
# 读取图像
image = cv2.imread('lane_image.jpg')
```
2. 转换图像为灰度图像
```python
gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
```
3. 进行高斯模糊处理以平滑图像
```python
kernel_size = 5
blur_gray = cv2.GaussianBlur(gray, (kernel_size, kernel_size), 0)
```
4. 进行Canny边缘检测
```python
low_threshold = 50
high_threshold = 150
edges = cv2.Canny(blur_gray, low_threshold, high_threshold)
```
5. 创建一个ROI(感兴趣区域)来减少计算量
```python
mask = np.zeros_like(edges)
ignore_mask_color = 255
# 定义一个四边形的顶点
imshape = image.shape
vertices = np.array([[(0, imshape[0]), (450, 320), (550, 320), (imshape[1], imshape[0])]], dtype=np.int32)
# 将ROI区域填充为白色
cv2.fillPoly(mask, vertices, ignore_mask_color)
# 对ROI区域内的边缘进行与运算
masked_edges = cv2.bitwise_and(edges, mask)
```
6. 进行霍夫变换检测直线
```python
# 设置霍夫变换参数
rho = 2
theta = np.pi / 180
threshold = 20
min_line_length = 20
max_line_gap = 300
# 进行霍夫变换
lines = cv2.HoughLinesP(masked_edges, rho, theta, threshold, np.array([]), min_line_length, max_line_gap)
# 画出检测到的直线
line_image = np.zeros((masked_edges.shape[0], masked_edges.shape[1], 3), dtype=np.uint8)
for line in lines:
for x1, y1, x2, y2 in line:
cv2.line(line_image, (x1, y1), (x2, y2), (0, 0, 255), 10)
# 将线条和原始图像混合在一起
result = cv2.addWeighted(image, 0.8, line_image, 1, 0)
```
以上就是一个基于OpenCV的车道线检测的详细步骤。需要注意的是,该算法对于光线、天气等因素比较敏感,需要进行一定的调参和优化才能达到最佳效果。
相关推荐
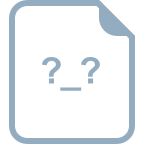
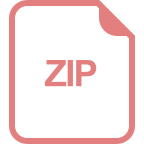














