用这个代码Rect rect = DetectRectangle(canny_Image, out points);实现
时间: 2023-06-24 13:02:59 浏览: 69
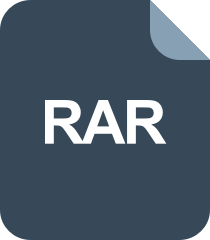
通过canny算子对图像进行边缘检测

这段代码的作用是利用Canny算法检测图像中的矩形,并返回四个点的坐标。其中,canny_Image是经过Canny算法处理后的图像。可以参考以下示例代码:
```csharp
// 读取图像
Mat image = Cv2.ImRead("test.jpg");
// 灰度化
Mat gray = new Mat();
Cv2.CvtColor(image, gray, ColorConversionCodes.BGR2GRAY);
// 边缘检测
Mat edges = new Mat();
Cv2.Canny(gray, edges, 50, 200);
// 检测矩形
Point[] points;
Rect rect = DetectRectangle(edges, out points);
// 在图像上绘制矩形
Cv2.Polylines(image, new Point[][] { points }, true, Scalar.Red);
// 显示图像
Cv2.ImShow("result", image);
Cv2.WaitKey();
```
其中,DetectRectangle函数的实现可以参考以下代码:
```csharp
public static Rect DetectRectangle(Mat image, out Point[] points)
{
// 轮廓检测
Point[][] contours;
HierarchyIndex[] hierarchy;
Cv2.FindContours(image, out contours, out hierarchy, RetrievalModes.External, ContourApproximationModes.ApproxSimple);
// 筛选矩形
List<Point[]> rectangles = new List<Point[]>();
for (int i = 0; i < contours.Length; i++)
{
Point[] approxCurve = Cv2.ApproxPolyDP(contours[i], Cv2.ArcLength(contours[i], true) * 0.02, true);
if (approxCurve.Length == 4 && Cv2.IsContourConvex(approxCurve))
{
double cos = Math.Abs(Cv2.ContourArea(approxCurve)) / (Cv2.ArcLength(approxCurve, true) * Cv2.ArcLength(approxCurve, true));
if (cos < 0.1)
{
rectangles.Add(approxCurve);
}
}
}
// 计算四边形的重心
Point[] centerPoints = new Point[rectangles.Count];
for (int i = 0; i < rectangles.Count; i++)
{
centerPoints[i] = new Point((rectangles[i][0].X + rectangles[i][2].X) / 2, (rectangles[i][0].Y + rectangles[i][2].Y) / 2);
}
// 根据距离筛选最大的矩形
Point centerPoint = new Point(image.Cols / 2, image.Rows / 2);
double maxDistance = 0;
Point[] maxRectangle = null;
for (int i = 0; i < rectangles.Count; i++)
{
double distance = Math.Sqrt((centerPoints[i].X - centerPoint.X) * (centerPoints[i].X - centerPoint.X) + (centerPoints[i].Y - centerPoint.Y) * (centerPoints[i].Y - centerPoint.Y));
if (distance > maxDistance)
{
maxDistance = distance;
maxRectangle = rectangles[i];
}
}
// 返回矩形四个点的坐标
points = maxRectangle;
return Cv2.BoundingRect(maxRectangle);
}
```
这个函数使用了轮廓检测和多边形拟合的方法,可以有效地检测图像中的矩形。
阅读全文
相关推荐
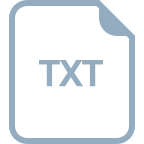
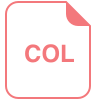
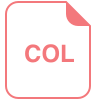












