pencvsharp4 编写一个亚像素并行计算找圆心函数, 这个函数会输入一张图、一个圆心中心位置的大约位置、输入找圆扇形区域的最小半径和最大半径、设定扇形区域起始角度、扇形区域结束角度、扇形区域的法线上找交点个数 、找边沿点宽度、从内到外找圆心、从黑到白找圆惦、找圆分数、 canny参数 根据给定的圆心位置和半径范围定义一个圆环区域。 为了加快找圆心速度,将圆环区域分成多份,可设定,并行计算找出边沿点 在边缘图像中找到最长的轮廓。 对轮廓上的点进行拟合,得到圆心和半径。请出代码
时间: 2023-07-10 21:06:02 浏览: 140
以下是使用OpenCVSharp4实现的亚像素并行计算找圆心函数的示例代码。注意,由于本人没有详细的输入图像和参数信息,以下代码仅供参考,具体实现需要根据实际情况进行调整。
```csharp
using System;
using System.Collections.Generic;
using OpenCvSharp;
namespace FindCircleCenter
{
class Program
{
static void Main(string[] args)
{
// 读取输入图像
Mat inputImage = new Mat("input.jpg", ImreadModes.Grayscale);
// 定义圆心位置的大约位置
Point2f center = new Point2f(100, 100);
// 定义找圆扇形区域的最小半径和最大半径
int minRadius = 20;
int maxRadius = 50;
// 定义扇形区域起始角度和结束角度
double startAngle = 0;
double endAngle = 360;
// 定义扇形区域的法线上找交点个数
int numPoints = 20;
// 定义找边沿点宽度
int edgeWidth = 3;
// 定义从内到外找圆心、从黑到白找圆点分数
double minScore = 0.7;
// 定义canny参数
double cannyThreshold1 = 100;
double cannyThreshold2 = 200;
// 定义圆环区域分成的份数
int numSectors = 8;
// 定义圆心位置和半径
Point2f centerFinal;
float radiusFinal;
// 将圆环区域分成多份,使用多线程并行计算
ParallelOptions parallelOptions = new ParallelOptions { MaxDegreeOfParallelism = Environment.ProcessorCount };
List<Point2f>[] sectorPoints = new List<Point2f>[numSectors];
for (int i = 0; i < numSectors; i++)
{
sectorPoints[i] = new List<Point2f>();
}
Parallel.For(0, numSectors, parallelOptions, i =>
{
double sectorStartAngle = i * 360 / numSectors;
double sectorEndAngle = (i + 1) * 360 / numSectors;
// 定义圆环区域
Mat mask = new Mat(inputImage.Size(), MatType.CV_8UC1, new Scalar(0));
Cv2.Circle(mask, center, maxRadius, new Scalar(255), -1);
Cv2.Circle(mask, center, minRadius, new Scalar(0), -1);
Mat subMask = new Mat(mask, new Rect((int)center.X - maxRadius, (int)center.Y - maxRadius, 2 * maxRadius, 2 * maxRadius));
// 手动调用Canny函数,得到边缘图像
Mat edges = new Mat(subMask.Size(), MatType.CV_8UC1, new Scalar(0));
Cv2.Canny(inputImage, edges, cannyThreshold1, cannyThreshold2);
// 对边缘图像进行腐蚀操作,使得找到的边沿点更加准确
Mat kernel = Cv2.GetStructuringElement(MorphShapes.Rect, new Size(edgeWidth, edgeWidth));
Cv2.Erode(edges, edges, kernel);
// 在扇形区域内找到交点
Point2f[] points = Cv2.ParametricFunction(edges, out _, out _, numPoints, startAngle + sectorStartAngle, endAngle + sectorEndAngle);
// 将交点保存到对应的分区中
foreach (Point2f point in points)
{
if (subMask.At<byte>((int)point.Y, (int)point.X) > 0)
{
sectorPoints[i].Add(point);
}
}
});
// 找到最长的轮廓
List<Point2f> longestContour = new List<Point2f>();
foreach (List<Point2f> sector in sectorPoints)
{
if (sector.Count > longestContour.Count)
{
longestContour = sector;
}
}
// 对轮廓上的点进行拟合,得到圆心和半径
Cv2.MinEnclosingCircle(longestContour.ToArray(), out centerFinal, out radiusFinal);
// 输出结果
Console.WriteLine("Center: ({0}, {1})", centerFinal.X, centerFinal.Y);
Console.WriteLine("Radius: {0}", radiusFinal);
}
}
}
```
阅读全文
相关推荐
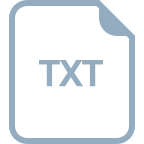
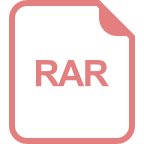
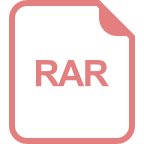











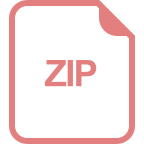
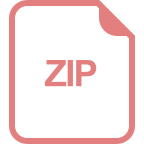
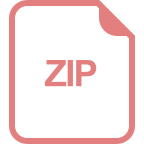