c# 向量处理几何图形代码
时间: 2023-07-27 17:31:28 浏览: 71
以下是一个简单的 C# 向量处理几何图形的代码示例:
```csharp
using System;
public struct Vector2D
{
public double x;
public double y;
public Vector2D(double x, double y)
{
this.x = x;
this.y = y;
}
public static Vector2D operator +(Vector2D v1, Vector2D v2)
{
return new Vector2D(v1.x + v2.x, v1.y + v2.y);
}
public static Vector2D operator -(Vector2D v1, Vector2D v2)
{
return new Vector2D(v1.x - v2.x, v1.y - v2.y);
}
public static double Dot(Vector2D v1, Vector2D v2)
{
return v1.x * v2.x + v1.y * v2.y;
}
public static double Cross(Vector2D v1, Vector2D v2)
{
return v1.x * v2.y - v1.y * v2.x;
}
public static double Magnitude(Vector2D v)
{
return Math.Sqrt(v.x * v.x + v.y * v.y);
}
public static Vector2D Normalize(Vector2D v)
{
double magnitude = Magnitude(v);
return new Vector2D(v.x / magnitude, v.y / magnitude);
}
public static double Distance(Vector2D v1, Vector2D v2)
{
double dx = v2.x - v1.x;
double dy = v2.y - v1.y;
return Math.Sqrt(dx * dx + dy * dy);
}
}
public class Program
{
public static void Main(string[] args)
{
// 创建两个向量
Vector2D v1 = new Vector2D(1, 2);
Vector2D v2 = new Vector2D(3, 4);
// 求和
Vector2D v3 = v1 + v2;
// 求差
Vector2D v4 = v2 - v1;
// 点积
double dot = Vector2D.Dot(v1, v2);
// 叉积
double cross = Vector2D.Cross(v1, v2);
// 向量长度
double magnitude = Vector2D.Magnitude(v1);
// 向量归一化
Vector2D v5 = Vector2D.Normalize(v1);
// 两向量间距离
double distance = Vector2D.Distance(v1, v2);
// 输出结果
Console.WriteLine("v3 = ({0}, {1})", v3.x, v3.y);
Console.WriteLine("v4 = ({0}, {1})", v4.x, v4.y);
Console.WriteLine("dot = {0}", dot);
Console.WriteLine("cross = {0}", cross);
Console.WriteLine("magnitude = {0}", magnitude);
Console.WriteLine("v5 = ({0}, {1})", v5.x, v5.y);
Console.WriteLine("distance = {0}", distance);
}
}
```
这个代码示例定义了一个名为 `Vector2D` 的结构体,其中包含了向量的各种操作,包括向量的加减、点积、叉积、长度、归一化和距离等。在 `Main` 函数中,我们使用这些操作来对两个向量进行处理,并输出结果。
阅读全文
相关推荐
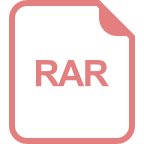
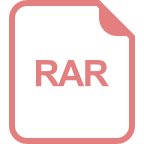
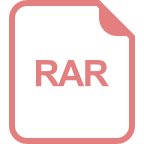
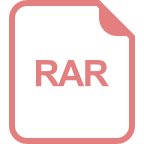
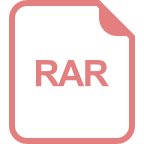
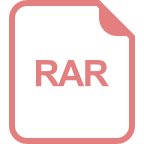
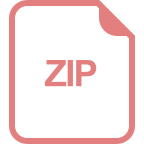
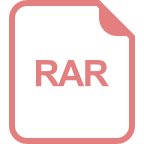
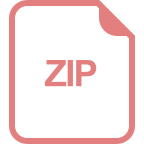
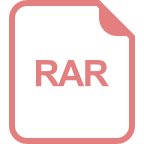
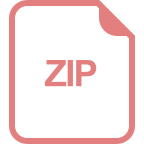
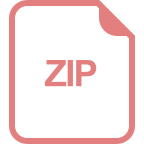
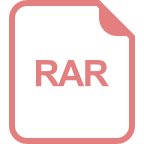
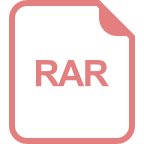
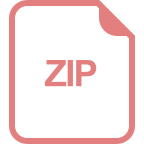
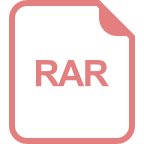