一段英文单词由若干单词组成,编写程序提取其中的所有单词。请实现由用 户输入英文,利用字符串存储英文,提取后的单词用向量容器存储。C++语言
时间: 2024-05-13 16:15:48 浏览: 179
以下是一个简单的 C++ 代码示例,可以实现从用户输入的一段英文中提取单词,并将其存储在向量容器中。
```c++
#include <iostream>
#include <string>
#include <vector>
using namespace std;
vector<string> extractWords(string str) {
vector<string> words;
string word = "";
for (int i = 0; i < str.length(); i++) {
// 如果当前字符是字母,则将其添加到单词中
if (isalpha(str[i])) {
word += str[i];
}
// 如果当前字符不是字母,且单词不为空,则将其添加到向量容器中
else if (word != "") {
words.push_back(word);
word = "";
}
}
// 如果最后一个单词不为空,则将其添加到向量容器中
if (word != "") {
words.push_back(word);
}
return words;
}
int main() {
string input;
cout << "请输入一段英文:";
getline(cin, input);
vector<string> words = extractWords(input);
cout << "提取出的单词有:" << endl;
for (int i = 0; i < words.size(); i++) {
cout << words[i] << endl;
}
return 0;
}
```
该程序首先定义了一个 `extractWords` 函数,该函数接受一个字符串参数,用于存储用户输入的英文,然后遍历该字符串中的每个字符,如果当前字符是字母,则将其添加到 `word` 变量中,直到遇到非字母字符。在遇到非字母字符时,如果 `word` 不为空,则将其添加到向量容器 `words` 中,然后将 `word` 重置为空字符串。最后,如果最后一个单词不为空,则将其添加到向量容器中。
在 `main` 函数中,该程序首先提示用户输入一段英文,并使用 `getline` 函数读取用户的输入。然后调用 `extractWords` 函数提取单词,并将提取出的单词按顺序输出到控制台上。
阅读全文
相关推荐
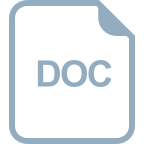
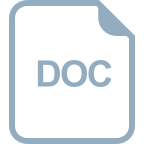
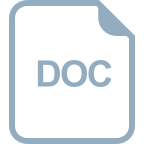











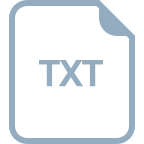
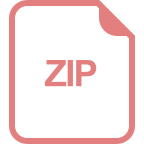
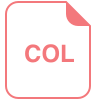
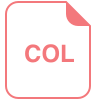
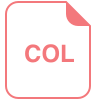