<?xml version="1.0" encoding="UTF-8"?> -<androidx.constraintlayout.widget.ConstraintLayout tools:context=".MainActivity" android:layout_height="match_parent" android:layout_width="match_parent" xmlns:tools="http://schemas.android.com/tools" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:android="http://schemas.android.com/apk/res/android"> <androidx.fragment.app.FragmentContainerView android:layout_height="match_parent" android:layout_width="match_parent" app:navGraph="@navigation/nav_graph" app:layout_constraintVertical_bias="0.452" app:layout_constraintTop_toTopOf="parent" app:layout_constraintStart_toStartOf="parent" app:layout_constraintHorizontal_bias="0.692" app:layout_constraintEnd_toEndOf="parent" app:layout_constraintBottom_toBottomOf="parent" app:defaultNavHost="true" android:name="androidx.navigation.fragment.NavHostFragment" android:id="@+id/fragmentContainerView"/> </androidx.constraintlayout.widget.ConstraintLayout>
时间: 2023-12-05 12:03:42 浏览: 50
这段代码是一个使用了 ConstraintLayout 布局的 Android 应用程序中的布局文件,其中包含了一个 FragmentContainerView,用于承载一个导航图(nav_graph),并且设置了该 FragmentContainerView 为默认的导航宿主(defaultNavHost)。同时,该布局文件还使用了 app 命名空间和 tools 命名空间,分别代表了 application 和 design-time tools。其中 app:navGraph 属性用于设置导航图,而 app:layout_constraint* 属性用于设置视图的约束条件,这些约束条件控制了视图的位置和大小。
相关问题
<?xml version="1.0" encoding="utf-8"?> <androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" tools:context=".MainActivity"> <TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Hello !" app:layout_constraintBottom_toBottomOf="parent" app:layout_constraintEnd_toEndOf="parent" app:layout_constraintHorizontal_bias="0.446" app:layout_constraintStart_toStartOf="parent" app:layout_constraintTop_toTopOf="parent" /> </androidx.constraintlayout.widget.ConstraintLayout>解释这段代码
这是一个简单的布局文件的代码段,使用的是Android的约束布局(ConstraintLayout)。
在布局文件中,只有一个TextView控件,其宽度和高度都设置为wrap_content,即根据文本内容自适应大小。文本内容为“Hello !”。
使用约束布局时,需要为每个控件指定其相对于其他控件或父布局的约束条件。在这个例子中,TextView的约束条件为:
- app:layout_constraintBottom_toBottomOf="parent",即TextView的底部与父布局的底部对齐。
- app:layout_constraintEnd_toEndOf="parent",即TextView的右侧与父布局的右侧对齐。
- app:layout_constraintHorizontal_bias="0.446",即水平方向上TextView在父布局中的位置偏移量为0.446。
- app:layout_constraintStart_toStartOf="parent",即TextView的左侧与父布局的左侧对齐。
- app:layout_constraintTop_toTopOf="parent",即TextView的顶部与父布局的顶部对齐。
这些约束条件可以确保TextView始终位于父布局的中心位置。
怎么在activity_main.xml中设计登录和注册页面的UI,可以使用EditText、Button等控件实现
在activity_main.xml文件中,使用以下代码可以创建一个简单的登录和注册页面的UI界面,包含用户名、密码、登录和注册两个按钮:
```
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<EditText
android:id="@+id/usernameEditText"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="48dp"
android:layout_marginTop="64dp"
android:layout_marginEnd="48dp"
android:hint="请输入用户名"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<EditText
android:id="@+id/passwordEditText"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="48dp"
android:layout_marginTop="16dp"
android:layout_marginEnd="48dp"
android:hint="请输入密码"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/usernameEditText" />
<Button
android:id="@+id/loginButton"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="32dp"
android:text="登录"
app:layout_constraintEnd_toEndOf="@+id/passwordEditText"
app:layout_constraintStart_toStartOf="@+id/passwordEditText"
app:layout_constraintTop_toBottomOf="@+id/passwordEditText" />
<Button
android:id="@+id/registerButton"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="16dp"
android:text="注册"
app:layout_constraintEnd_toEndOf="@+id/loginButton"
app:layout_constraintStart_toStartOf="@+id/loginButton"
app:layout_constraintTop_toBottomOf="@+id/loginButton" />
</androidx.constraintlayout.widget.ConstraintLayout>
```
在这个布局文件中,我们使用了ConstraintLayout布局容器,通过约束来定义控件的位置和大小。其中,EditText控件用于输入用户名和密码,Button控件用于登录和注册操作。
相关推荐
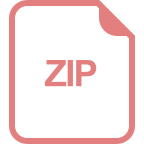
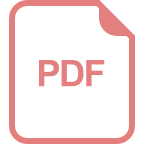
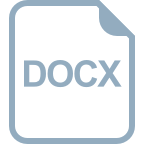












