python字典值抽取
时间: 2024-05-23 15:08:03 浏览: 15
Python字典是一种用于存储键值对的数据结构,可以通过键来访问对应的值。如果你想要从字典中抽取值,可以使用字典的values()方法,该方法返回一个包含字典中所有值的列表。示例代码如下:
```
my_dict = {'a': 1, 'b': 2, 'c': 3}
values_list = list(my_dict.values())
print(values_list)
```
输出结果为:
```
[1, 2, 3]
```
相关问题
python字典键值抽取
Python字典是一种无序的数据结构,它由一系列键和相应的值组成。如果想要从字典中提取键或值,可以使用字典提供的方法。
提取字典的键:
可以使用keys()方法获取所有键,也可以使用for循环遍历字典并逐个提取键。例如:
```
my_dict = {'name': 'Tom', 'age': 18, 'gender': 'male'}
# 获取所有键
keys = my_dict.keys()
print(keys) # dict_keys(['name', 'age', 'gender'])
# 遍历字典并逐个提取键
for key in my_dict:
print(key)
```
提取字典的值:
可以使用values()方法获取所有值,也可以通过键来获取相应的值。例如:
```
my_dict = {'name': 'Tom', 'age': 18, 'gender': 'male'}
# 获取所有值
values = my_dict.values()
print(values) # dict_values(['Tom', 18, 'male'])
# 通过键来获取相应的值
age = my_dict['age']
print(age) # 18
```
使用random随机抽取字典中的值
好的,可以使用Python中的random模块来随机抽取字典中的值。具体操作可以参考以下代码:
```python
import random
my_dict = {"a": 1, "b": 2, "c": 3, "d": 4}
random_key = random.choice(list(my_dict.keys()))
random_value = my_dict[random_key]
print("随机抽取的键为: ", random_key)
print("对应的值为: ", random_value)
```
这里使用了random模块中的choice函数来随机选择字典中的一个键,然后再利用这个键来获取对应的值。注意,由于字典是无序的,所以需要先将字典的键转化为列表,才能进行随机选择。
相关推荐
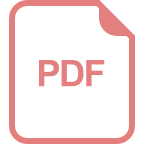
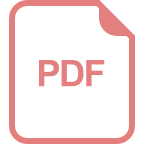
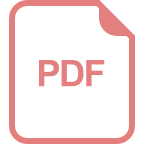












