稀疏矩阵三元组计算器
时间: 2024-12-24 21:10:37 浏览: 8
稀疏矩阵是一种在计算机科学中常用的矩阵数据结构,其中大部分元素都是零。在处理这类矩阵时,用三元组表示更为高效,因为非零元素及其对应的值通常只需要三个属性(行索引、列索引和数值)来存储,而不是传统的二维数组那样。
一个简单的稀疏矩阵三元组计算器可能包含以下功能:
1. **插入元素**:接收行、列和值,如果这个位置不在矩阵中,则创建新条目;如果已经在矩阵中,更新现有值。
```cpp
struct SparseMatrixTriple {
int row;
int col;
double value;
};
void insert(SparseMatrixTriple triple, std::unordered_map<std::pair<int, int>, double>& matrix) {
if (matrix.find(triple.row) == matrix.end() || matrix[triple.row].find(triple.col) == matrix[triple.row].end()) {
matrix[triple.row][triple.col] = triple.value;
} else {
matrix[triple.row][triple.col] += triple.value;
}
}
```
2. **查找元素**:根据给定的行和列快速找到对应的值,如果不存在则返回0或抛异常。
```cpp
double get(int row, int col, const std::unordered_map<std::pair<int, int>, double>& matrix) {
auto it = matrix.find(row);
if (it != matrix.end() && it->second.find(col) != it->second.end()) {
return it->second[col];
}
return 0; // 或者抛异常
}
```
3. **计算矩阵操作**:如加法、乘法等,可能会涉及到遍历并处理稀疏矩阵中的所有非零元素。
```cpp
// 示例,假设我们有两个稀疏矩阵的三元组表示
std::vector<SparseMatrixTriple> mat1, mat2;
// 加法运算
for (const auto& t : mat1) {
insert(t, result);
}
// 乘法运算(这里仅示例,实际实现更复杂)
for (const auto& t1 : mat1) {
for (auto& t2 : mat2) {
if (t1.row == t2.col) { // 需要考虑稀疏矩阵的对角线规则
SparseMatrixTriple newTriple{t1.row, t2.row, t1.value * t2.value};
insert(newTriple, result);
}
}
}
```
阅读全文
相关推荐





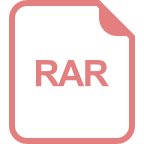




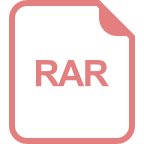


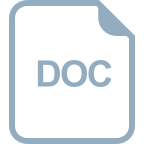



