编写一个Pthreads程序实现一个“任务队列”。主线程启动用户指定数量的线程,这些线程进入条件等待状态。主线程生成一些任务(一定计算量),每生成一个新的任务,就用条件变量唤醒一个线程,当这个唤醒线程执行完任务时,回到条件等待状态。当主线程生成完所有任务,设置全局变量表示再没有要生成的任务了,并用一个广播唤醒所有线程。为了清晰起见,建议任务采用链表操作
时间: 2024-04-12 15:31:01 浏览: 28
下面是一个使用Pthreads编写的任务队列程序示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <pthread.h>
typedef struct Node {
int data;
struct Node* next;
} Node;
typedef struct Queue {
Node* front;
Node* rear;
} Queue;
Queue taskQueue;
pthread_mutex_t queueMutex = PTHREAD_MUTEX_INITIALIZER;
pthread_cond_t queueCond = PTHREAD_COND_INITIALIZER;
int taskCount = 0;
int numThreads;
void enqueue(Queue* queue, int data) {
Node* newNode = (Node*)malloc(sizeof(Node));
newNode->data = data;
newNode->next = NULL;
if (queue->front == NULL) {
queue->front = newNode;
queue->rear = newNode;
} else {
queue->rear->next = newNode;
queue->rear = newNode;
}
}
int dequeue(Queue* queue) {
if (queue->front == NULL) {
return -1; // 队列为空
}
int data = queue->front->data;
Node* temp = queue->front;
if (queue->front == queue->rear) {
queue->front = NULL;
queue->rear = NULL;
} else {
queue->front = queue->front->next;
}
free(temp);
return data;
}
void* workerThread(void* arg) {
int threadId = *(int*)arg;
while (1) {
pthread_mutex_lock(&queueMutex);
while (taskCount == 0) {
pthread_cond_wait(&queueCond, &queueMutex);
}
int task = dequeue(&taskQueue);
taskCount--;
pthread_mutex_unlock(&queueMutex);
// 执行任务
printf("Thread %d executing task: %d\n", threadId, task);
// 模拟任务执行时间
usleep(rand() % 1000000);
if (taskCount == 0) {
pthread_cond_broadcast(&queueCond);
break;
}
}
printf("Thread %d finished\n", threadId);
return NULL;
}
int main() {
int i;
// 初始化任务队列
taskQueue.front = NULL;
taskQueue.rear = NULL;
printf("Enter the number of threads: ");
scanf("%d", &numThreads);
pthread_t* threads = (pthread_t*)malloc(numThreads * sizeof(pthread_t));
int* threadIds = (int*)malloc(numThreads * sizeof(int));
// 创建线程
for (i = 0; i < numThreads; i++) {
threadIds[i] = i;
pthread_create(&threads[i], NULL, workerThread, &threadIds[i]);
}
// 生成任务并加入队列
for (i = 0; i < 10; i++) {
pthread_mutex_lock(&queueMutex);
enqueue(&taskQueue, i);
taskCount++;
pthread_cond_signal(&queueCond);
pthread_mutex_unlock(&queueMutex);
// 模拟任务生成时间
usleep(rand() % 1000000);
}
// 等待线程完成任务
for (i = 0; i < numThreads; i++) {
pthread_join(threads[i], NULL);
}
free(threads);
free(threadIds);
return 0;
}
```
在这个程序中,主线程通过条件变量(`queueCond`)和互斥量(`queueMutex`)与工作线程进行同步操作。主线程生成任务并将其加入任务队列中,然后通过条件变量唤醒一个等待的线程来执行任务。工作线程在完成任务后回到条件等待状态,直到主线程生成完所有任务并发出广播信号来唤醒所有线程。
注意:此程序仅为示例,可能需要根据实际需求进行修改和完善。
相关推荐
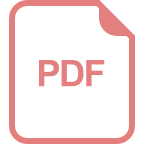
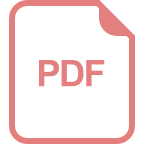
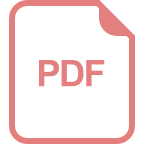















