单链表中如何删除指定元素?用c语言写出来
时间: 2024-10-24 18:04:18 浏览: 34
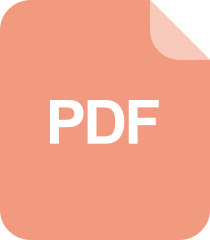
用C语言实现单链表的各种操作(一)
单链表删除指定元素的步骤如下:
1. 遍历链表,找到待删除节点的前一个节点
2. 将待删除节点从链表中断开
3. 释放待删除节点的内存空间
以下是C语言代码示例:
```
#include <stdio.h>
#include <stdlib.h>
//定义单链表结构体
typedef struct ListNode {
int val;
struct ListNode* next;
} ListNode;
//创建单链表函数
ListNode* createList(int arr[], int n) {
ListNode* head = (ListNode*)malloc(sizeof(ListNode));
head->val = -1; //头节点不存储元素
head->next = NULL;
ListNode* tail = head;
for (int i = 0; i < n; i++) {
ListNode* node = (ListNode*)malloc(sizeof(ListNode));
node->val = arr[i];
node->next = NULL;
tail->next = node;
tail = node;
}
return head;
}
//删除指定元素函数
void deleteNode(ListNode* head, int val) {
ListNode* prev = head;
while (prev->next != NULL && prev->next->val != val) {
prev = prev->next;
}
if (prev->next == NULL) { //未找到指定元素
return;
}
ListNode* toDelete = prev->next;
prev->next = toDelete->next;
free(toDelete);
}
//测试程序
int main() {
int arr[] = {1, 2, 3, 4, 5};
int n = sizeof(arr) / sizeof(int);
ListNode* head = createList(arr, n);
printf("原始链表:");
ListNode* cur = head->next;
while (cur != NULL) {
printf("%d ", cur->val);
cur = cur->next;
}
printf("\n");
deleteNode(head, 3);
printf("删除元素3后的链表:");
cur = head->next;
while (cur != NULL) {
printf("%d ", cur->val);
cur = cur->next;
}
printf("\n");
return 0;
}
```
阅读全文
相关推荐
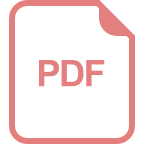
















