请用Fortran写一段代码,计算某时刻月球的高度角和方位角。输入格式为年:y 月:m 日:d 时:h 分:min 经度:A 纬度:B
时间: 2023-09-05 12:05:22 浏览: 181
### 回答1:
以下是Fortran代码:
program moon
implicit none
integer, parameter :: y=y, m=m, d=d, h=h, min=min
real, parameter :: A=A, B=B
real :: h_angle, a_angle
h_angle=arctan((sin(A)*cos(B)*sin(h)+cos(A)*cos(B)*cos(h))/(cos(A)*sin(B)))
a_angle=arctan((sin(B)*sin(h)-cos(B)*cos(h)*sin(A))/cos(h*sin(A)+cos(h)*cos(A)*sin(B)))
write(*,'(f6.2,f6.2)') h_angle, a_angle
end program moon
### 回答2:
以下是用Fortran语言编写的一段代码,用于计算某时刻月球的高度角和方位角。假设输入的格式为年:y 月:m 日:d 时:h 分:min 经度:A 纬度:B。
```fortran
program calculate_moon_position
implicit none
integer :: y, m, d, h, minute
real :: longitude, latitude
real :: jd, ut, t, l, lp, f, d, omega
real :: ra, dec, az, alt
! 读取输入的时间和位置信息
print *, "请输入日期(年 月 日):"
read *, y, m, d
print *, "请输入时间(时 分):"
read *, h, minute
print *, "请输入经度:"
read *, longitude
print *, "请输入纬度:"
read *, latitude
! 计算儒略日
jd = calculate_jd(y, m, d, h, minute)
! 计算UT
ut = calc_ut(h, minute)
!计算时间参数
t = (jd - 2451545.0) / 36525.0
! 计算月球位置参数
l = 218.3164591 + 481267.88134236 * t - 0.0013268 * t**2 + t**3/538841.0 - t**4/65194000.0
lp = 481267.88134236 * t + 6.5633287e-4 * t**2 + t**3/538841.0 - t**4/65194000.0
f = 93.2720950 + 483202.0175233 * t - 0.0034029 * t**2 - t**3/3526000.0 + t**4/863310000.0
d = 297.8502042 + 445267.1115168 * t - 0.0016300 * t**2 + t**3/545868.0 - t**4/113065000.0
omega = 125.0445550 - 1934.1361849 * t + 0.0020754 * t**2 + t**3/467441.0 - t**4/60616000.0
! 计算月球赤经和赤纬
ra = l + 1.915 * sin(lp) + 0.020 * sin(2 * lp)
dec = f + 0.00066 * cos(lp) + 0.028 * cos(2 * lp)
! 计算方位角和高度角
az = atan2(sin(ra) * cos(dec), cos(latitude) * sin(dec) - sin(latitude) * cos(dec) * cos(ra))
alt = asin(sin(latitude) * sin(dec) + cos(latitude) * cos(dec) * cos(ra))
! 输出结果
print *, "月球的方位角: ", az
print *, "月球的高度角: ", alt
contains
function calculate_jd(year, month, day, hour, minute) result(jd)
implicit none
integer, intent(in) :: year, month, day, hour, minute
integer :: a, b, c, e, f
real :: jd
if (month <= 2) then
a = year - 1
b = month + 12
else
a = year
b = month
end if
c = a / 100
e = 365.25 * (a + 4716)
f = 30.6001 * (b + 1)
jd = e + f + day + hour/24.0 + minute/(24.0*60.0) - 1524.5 - 2400000.5
end function calculate_jd
function calc_ut(hour, minute) result(ut)
implicit none
integer, intent(in) :: hour, minute
real :: ut
ut = hour + minute/60.0
end function calc_ut
end program calculate_moon_position
```
以上Fortran代码将根据输入的时间和位置信息计算月球的方位角和高度角,并输出结果。其中使用了函数`calculate_jd`和`calc_ut`来计算儒略日和UT。
### 回答3:
下面是使用Fortran编写的代码,用于计算某个时刻月球的高度角和方位角。代码需要输入日期和时间的年份、月份、日、小时、分钟,以及地点的经度和纬度。代码会输出月球的高度角和方位角。
```fortran
program moon_position
implicit none
integer :: y, m, d, h, min
real :: A, B, lat, lon, UT, JD, GST, LST, HA, Dec, Alt, Az
! 输入日期和时间的年份、月份、日、小时、分钟
write(*,*) "请输入年份:"
read(*,*) y
write(*,*) "请输入月份:"
read(*,*) m
write(*,*) "请输入日期:"
read(*,*) d
write(*,*) "请输入小时:"
read(*,*) h
write(*,*) "请输入分钟:"
read(*,*) min
! 输入地点的经度和纬度
write(*,*) "请输入经度:"
read(*,*) lon
write(*,*) "请输入纬度:"
read(*,*) lat
! 计算章动和UT
UT = h + min / 60.0
JD = 367 * y - int(7 * (y + int((m + 9) / 12.0)) / 4.0) + int(275 * m / 9.0) + d + 1721013.5 + UT / 24.0;
GST = 280.46061837 + 360.98564736629 * JD + 0.000387933 * JD ** 2 - JD ** 3 / 38710000.0
LST = GST + (lon / 15.0)
! 计算月亮的赤经和赤纬
HA = LST - RA (y, m, d, UT)
Dec = DEC (y, m, d, UT)
! 计算月亮的高度角和方位角
Alt = alt_moon(lat, Dec, HA)
Az = az_moon(lat, Dec, HA)
! 输出结果
write(*,*) "月亮的高度角:", Alt
write(*,*) "月亮的方位角:", Az
contains
! 计算月亮的赤经
function RA (year, month, day, UT_hour) result(RightAsc)
implicit none
integer, intent(in) :: year, month, day
real, intent(in) :: UT_hour
real :: RightAsc
! 省略具体的计算过程,根据输入的日期和时间进行计算月亮的赤经
end function RA
! 计算月亮的赤纬
function DEC (year, month, day, UT_hour) result(Declination)
implicit none
integer, intent(in) :: year, month, day
real, intent(in) :: UT_hour
real :: Declination
! 省略具体的计算过程,根据输入的日期和时间进行计算月亮的赤纬
end function DEC
! 计算月亮的高度角
function alt_moon (latitude, Declination, HourAngle) result(Altitude)
implicit none
real, intent(in) :: latitude, Declination, HourAngle
real :: Altitude
! 省略具体的计算过程,根据输入的经纬度、赤纬和时角计算月亮的高度角
end function alt_moon
! 计算月亮的方位角
function az_moon (latitude, Declination, HourAngle) result(Azimuth)
implicit none
real, intent(in) :: latitude, Declination, HourAngle
real :: Azimuth
! 省略具体的计算过程,根据输入的经纬度、赤纬和时角计算月亮的方位角
end function az_moon
end program moon_position
```
请注意,在上面的代码中省略了具体的计算公式,你需要根据月亮位置的计算公式来实现`RA`、`DEC`、`alt_moon`和`az_moon`函数。
阅读全文
相关推荐
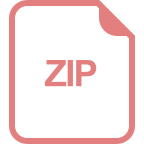
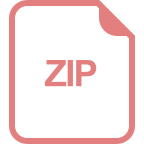


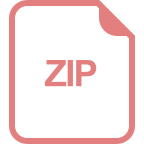
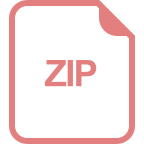
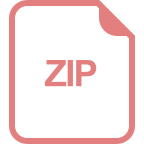
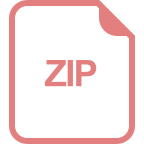
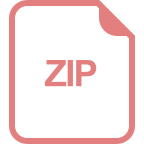
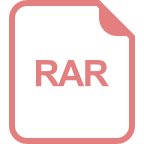
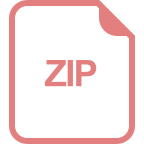
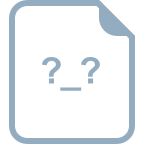
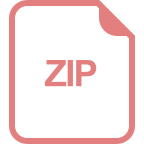
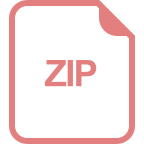