python 安卓xml.etree
时间: 2024-05-10 18:17:11 浏览: 143
如果您想在 Python 中处理 Android 的 XML 文件,可以使用 Python 的 xml.etree.ElementTree 模块。这个模块提供了一种方便的方式来解析 XML 文件并以树状结构的形式表示它们。
以下是一个示例代码,它使用 xml.etree.ElementTree 模块从一个 Android XML 文件中读取数据:
```python
import xml.etree.ElementTree as ET
# 打开 XML 文件并解析为树状结构
tree = ET.parse('path/to/your/android.xml')
root = tree.getroot()
# 遍历树状结构并提取数据
for child in root:
print(child.tag, child.attrib)
for subchild in child:
print(subchild.tag, subchild.attrib)
```
在这个示例中,您需要将 'path/to/your/android.xml' 替换为您实际的 Android XML 文件的路径。然后,使用 ET.parse() 函数将 XML 文件解析为树状结构,并使用 getroot() 方法获取树的根元素。接下来,您可以遍历树状结构并提取数据。
注意,xml.etree.ElementTree 模块只能处理符合 XML 规范的文件,如果您的 Android XML 文件格式不正确,将会导致解析错误。
相关问题
python 读取遍历手机xml
要读取和遍历手机XML,您可以使用Python的xml.etree.ElementTree模块。
以下是一个示例代码,读取Android手机上的contacts.xml文件:
```python
import xml.etree.ElementTree as ET
# 打开文件
tree = ET.parse('path/to/contacts.xml')
root = tree.getroot()
# 遍历所有联系人
for contact in root.findall('contact'):
# 获取联系人的名字
name = contact.find('name').text
# 获取联系人的电话号码
phone = contact.find('phone').text
# 打印联系人的信息
print(f'Name: {name}, Phone: {phone}')
```
在这个示例中,我们首先使用ET.parse()方法打开XML文件,然后使用getroot()方法获取XML树的根元素。接下来,我们使用findall()方法遍历所有contact元素,并使用find()方法获取每个联系人的名字和电话号码。
您可以根据需要修改代码来适应您的XML文件格式和数据结构。
Python解析uiautomator dump /sdcard/ui.xml
Python解析uiautomator dump生成的XML文件可以使用xml.etree.ElementTree模块。以下是一个示例代码,演示如何打印UI层次结构:
```python
import xml.etree.ElementTree as ET
# 加载XML文件
tree = ET.parse('/sdcard/ui.xml')
root = tree.getroot()
# 打印UI层次结构
def print_node(node, indent=''):
print(indent + node.tag)
for child in node:
print_node(child, indent + ' ')
print_node(root)
```
这将输出类似以下的UI层次结构:
```
hierarchy
android.widget.FrameLayout
android.widget.LinearLayout
android.widget.TextView
android.widget.LinearLayout
android.widget.TextView
android.widget.ImageView
android.widget.ScrollView
android.widget.LinearLayout
android.widget.LinearLayout
android.widget.TextView
android.widget.EditText
android.widget.TextView
android.widget.Button
```
您可以根据自己的需要修改代码,以便解析和提取特定的UI元素。
阅读全文
相关推荐
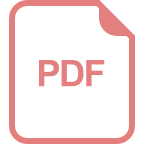
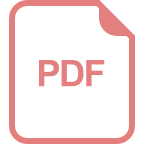
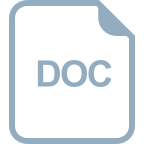
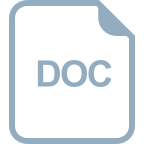
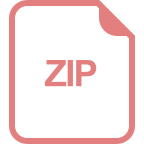
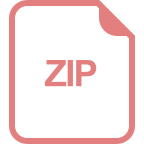
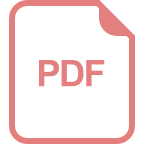
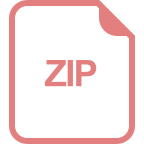
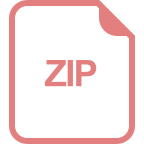
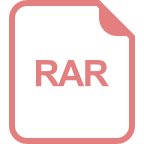
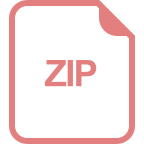
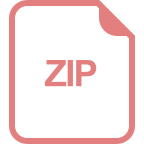
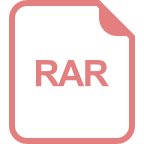
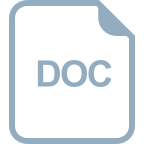
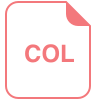
