c++ vector数组存放元素为char数组 如何向vector数组中添加元素 使char数组元素顺序不变
时间: 2023-12-23 17:05:15 浏览: 51
如果要向一个存放 char 数组的 vector 中添加一个新的元素,并且保持 char 数组中元素的顺序不变,你可以使用以下代码:
```c++
#include <iostream>
#include <vector>
#include <cstring>
using namespace std;
int main()
{
// 定义 vector 存放 char 数组
vector<char*> v;
// 定义一个新的 char 数组
char str[] = "hello world";
// 在 vector 末尾添加一个新的 char 数组
v.push_back(strdup(str));
// 输出 vector 中的元素
for (int i = 0; i < v.size(); i++) {
cout << v[i] << endl;
}
return 0;
}
```
这里使用了 `strdup()` 函数,它会为新的 char 数组在堆上分配内存,并将原来的字符串复制到新的内存空间中。这样做的好处是,如果你在程序中修改了原来的字符串,新的 char 数组不会受到影响。
相关问题
c++ 使用迭代器遍历 vector数组中的 char数组 并取出每一个char数组的值
可以使用如下代码来实现:
```c++
#include <iostream>
#include <vector>
int main() {
std::vector<char*> charVector{"hello", "world", "!"};
for (auto it = charVector.begin(); it != charVector.end(); ++it) {
char* currentChar = *it;
for (int i = 0; currentChar[i] != '\0'; ++i) {
std::cout << currentChar[i] << " ";
}
std::cout << std::endl;
}
return 0;
}
```
在这个例子中,我们创建了一个 `std::vector<char*>`,其中每个元素都是一个 char 指针(即一个 char 数组)。然后我们使用迭代器 `it` 遍历这个 vector,每次取出一个 char 指针,并使用一个 `for` 循环遍历这个 char 数组中的每个 char,直到遇到 `\0` 结束符。最后,我们输出每个 char 的值并换行。
c语言将char数组插入vector<char>里,相同的内容不插入
可以使用循环遍历char数组,然后逐个判断是否已经存在于vector中,如果不存在则将其插入。可以参考以下代码:
```c++
#include <iostream>
#include <vector>
#include <cstring>
using namespace std;
int main() {
char arr[] = "hello world";
vector<char> vec;
for (int i = 0; i < strlen(arr); i++) {
bool exist = false;
for (int j = 0; j < vec.size(); j++) {
if (arr[i] == vec[j]) {
exist = true;
break;
}
}
if (!exist) {
vec.push_back(arr[i]);
}
}
for (int i = 0; i < vec.size(); i++) {
cout << vec[i];
}
cout << endl;
return 0;
}
```
输出结果为:
```
helo wrd
```
其中,`exist`变量表示当前字符是否已经存在于vector中,如果存在则将其标记为`true`,否则标记为`false`。在循环遍历完当前字符之后,如果`exist`为`false`,则将其插入到vector中。最后,使用循环遍历vector,将所有字符输出。
相关推荐
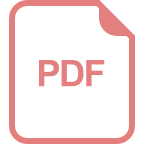
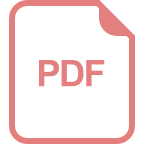












