c++的unordered_map
时间: 2023-10-13 11:20:08 浏览: 89
unordered_map是C++ STL中的关联容器之一,用于存储键值对,其中键和值都可以是任何数据类型。与map不同的是,unordered_map中的元素是无序的,因此查找和插入的时间复杂度平均为O(1),最坏情况下为O(n)。unordered_map的实现是基于哈希表的,因此它的查找和插入速度比map更快,但是它的内存占用也更大一些。因为哈希表需要额外的空间来存储哈希表本身以及每个桶中的链表或向量。unordered_map支持以下操作:
1. insert(key, value):插入一个键值对。
2. erase(key):删除指定键的元素。
3. find(key):查找指定键的元素。
4. size():返回元素个数。
5. empty():判断容器是否为空。
6. clear():清空容器中的所有元素。
下面是一个unordered_map的示例:
```c++
#include <iostream>
#include <unordered_map>
using namespace std;
int main() {
unordered_map<string, int> my_map;
my_map.insert(make_pair("apple", 3));
my_map.insert(make_pair("banana", 2));
my_map.insert(make_pair("orange", 5));
my_map["pear"] = 4;
cout << "my_map contains:";
for (auto it = my_map.begin(); it != my_map.end(); ++it)
cout << " " << it->first << ":" << it->second;
cout << endl;
my_map.erase("banana");
cout << "my_map contains:";
for (auto it = my_map.begin(); it != my_map.end(); ++it)
cout << " " << it->first << ":" << it->second;
cout << endl;
auto search = my_map.find("pear");
if (search != my_map.end())
cout << "Found " << search->first << " with value " << search->second << endl;
else
cout << "Key not found" << endl;
cout << "my_map size is " << my_map.size() << endl;
if (my_map.empty())
cout << "my_map is empty" << endl;
else
cout << "my_map is not empty" << endl;
my_map.clear();
if (my_map.empty())
cout << "my_map is empty" << endl;
else
cout << "my_map is not empty" << endl;
return 0;
}
```
输出结果为:
```
my_map contains: orange:5 apple:3 pear:4 banana:2
my_map contains: orange:5 apple:3 pear:4
Found pear with value 4
my_map size is 3
my_map is not empty
my_map is empty
```
阅读全文
相关推荐
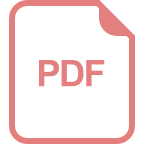
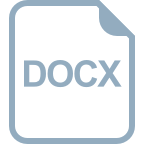















