帮我写个解密维吉尼亚密码的代码
时间: 2023-05-10 08:55:44 浏览: 92
好的,以下是一个简单的 Python 代码,可以解密维吉尼亚密码:
```python
def vigenere_decrypt(ciphertext, key):
plaintext = ""
key_len = len(key)
for i, c in enumerate(ciphertext):
shift = ord(key[i % key_len]) - ord('a')
if c.isupper():
plaintext += chr((ord(c) - shift - 65) % 26 + 65)
elif c.islower():
plaintext += chr((ord(c) - shift - 97) % 26 + 97)
else:
plaintext += c
return plaintext
```
你可以将密文和密钥作为参数传递给这个函数,它将返回解密后的明文。请注意,这个函数假设密文和密钥都是纯文本字符串,且密钥只包含小写字母。
如果你需要更复杂的维吉尼亚密码解密算法,可以在网上搜索更多的资源。
相关问题
维吉尼亚密码加解密c语言简单代码
维吉尼亚密码是一种基于字母替换的古典加密技术,通常需要一个称为“凯撒表”的密钥来进行加解密。下面是一个简单的C语言代码示例,展示了如何实现维吉尼亚密码的加解密过程:
```c
#include <stdio.h>
#include <string.h>
#define ALPHABET_SIZE 26
// 加密函数
char encrypt(char c, char key) {
if (c >= 'A' && c <= 'Z') { // 大写字母
return ((c - 'A' + key) % ALPHABET_SIZE) + 'A';
} else if (c >= 'a' && c <= 'z') { // 小写字母
return ((c - 'a' + key) % ALPHABET_SIZE) + 'a';
}
return c; // 非字母字符保持不变
}
// 解密函数
char decrypt(char c, char key) {
return encrypt(c, -key);
}
int main() {
char plaintext[100], ciphertext[100];
char key[ALPHABET_SIZE];
printf("请输入明文:\n");
fgets(plaintext, sizeof(plaintext), stdin);
printf("请输入密钥(大小写字母):\n");
fgets(key, sizeof(key), stdin);
key[strlen(key) - 1] = '\0'; // 去除换行符
// 对明文进行加密
for (int i = 0; plaintext[i]; ++i) {
ciphertext[i] = encrypt(plaintext[i], key[i]);
}
ciphertext[strlen(plaintext)] = '\0';
printf("加密后的密文为:\n%s\n", ciphertext);
// 如果需要,也可以在这里添加一个选项来直接解密
// char decrypted[] = ...;
// printf("解密后的明文为:\n%s\n", decrypted);
return 0;
}
```
这个程序首先提示用户输入明文和密钥,然后使用`encrypt`函数对每个字符进行加密,并打印出结果。如果你想实时解密,可以在代码里添加相应的部分。
维吉尼亚密码加密和解密的java代码
维吉尼亚密码(Vigenere Cipher)是一种古典的替换式密码,它使用一个简单的字母表替代模式来加密文本。这个模式通常是一个密钥(也可能是单词),重复应用于原文中的每个字符。以下是一个基本的Java代码实现维吉尼亚密码的加密和解密过程:
```java
import java.util.HashMap;
import java.util.Map;
public class VigenereCipher {
private static final char ALPHABET[] = "ABCDEFGHIJKLMNOPQRSTUVWXYZ";
// 加密函数
public static String encrypt(String plaintext, String key) {
int keyIndex = 0;
StringBuilder encryptedText = new StringBuilder();
for (char c : plaintext.toCharArray()) {
if (Character.isLetter(c)) {
int shift = ALPHABET.indexOf(key.charAt(keyIndex++ % key.length()));
char encryptedChar = (c + shift) % 26 + 'A';
encryptedText.append(encryptedChar);
} else {
encryptedText.append(c);
}
}
return encryptedText.toString();
}
// 解密函数
public static String decrypt(String ciphertext, String key) {
int keyIndex = 0;
StringBuilder decryptedText = new StringBuilder();
for (char c : ciphertext.toCharArray()) {
if (Character.isLetter(c)) {
int shift = -ALPHABET.indexOf(key.charAt(keyIndex++ % key.length()));
char decryptedChar = (c - shift + 26) % 26 + 'A';
decryptedText.append(decryptedChar);
} else {
decryptedText.append(c);
}
}
return decryptedText.toString();
}
// 示例用法
public static void main(String[] args) {
String plaintext = "SECRET MESSAGE";
String key = "KEYWORD";
System.out.println("Original Text: " + plaintext);
System.out.println("Encrypted Text: " + encrypt(plaintext, key));
System.out.println("Decrypted Text: " + decrypt(encrypt(plaintext, key), key));
}
}
```
在这个代码中,`encrypt`方法接收明文和密钥,对每个字母进行位移并替换为密文;`decrypt`方法则执行相反的操作,将密文恢复回原文。
阅读全文
相关推荐
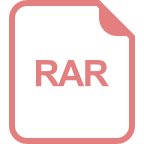
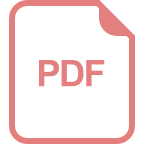
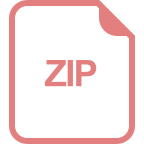
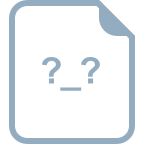
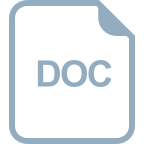




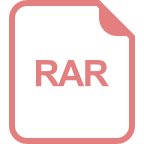




